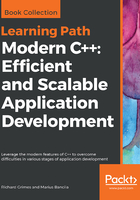
Arithmetic Operators
The arithmetic operators +, -, /, *, and % need little explanation other than perhaps the division and modulus operators. All of these operators act upon integer and real numeric types except for %, which can only be used with integer types. If you mix the types (say, add an integer to a floating-point number) then the compiler will perform an automatic conversion. The division operator / behaves as you expect for floating point variables: it produces the result of the division of the two operands. When you perform the division between two integers a / b, the result is the whole number of the divisor (b) in the dividend (a). The remainder of the division is obtained by the modulus %. So, for any integer, b (other than zero), one could say that, an integer a can be expressed as follows:
(a / b) * b + (a % b)
Note that the modulus operator can only be used with integers. If you want to get the remainder of a floating-point division, use the standard function, std:;remainder.
Be careful when using division with integers, since fractional parts are discarded. If you need the fractional parts, then you may need to explicitly convert the numbers into real numbers. For example:
int height = 480;
int width = 640;
float aspect_ratio = width / height;
This gives an aspect ratio of 1 when it should be 1.3333 (or 4 : 3). To ensure that floating-point division is performed, rather than integer division, you can cast either (or both) the dividend or divisor to a floating-point number.