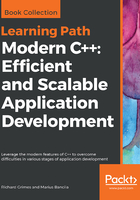
Determining the return type
Functions may be written to run a routine and not return a value. If this is the case, you must specify that the function returns void. In most cases, a function will return a value, if only to indicate that the function has completed correctly. There is no requirement that the calling function obtains the return value or does anything with it. The calling function can simply ignore the return value.
There are two ways to specify the return type. The first way is to give the type before the function name. This is the method used in most of the examples so far. The second way is called the trailing return type and requires that you place auto as the return type before the function name and use the -> syntax to give the actual return type after the parameter list:
inline auto mult(int lhs, int rhs) -> int
{
return lhs * rhs;
}
This function is so simple that it is a good candidate to be inlined. The return type on the left is given as auto, meaning that the actual return type is specified after the parameter list. The -> int means that the return type is int. This syntax has the same effect as using int on the left. This syntax is useful when a function is templated and the return type may not be noticeable.
In this trivial example, you can omit the return type entirely and just use auto on the left of the function name. This syntax means that the compiler will deduce the return type from the actual value returned. Clearly the compiler will only know what the return type is from the function body, so you cannot provide a prototype for such functions.
Finally, if a function does not return at all (for example, if it goes into a never-ending loop to poll some value) you can mark it with the C++11 attribute [[noreturn]]. The compiler can use this attribute to write more efficient code because it knows that it does not need to provide code to return a value.