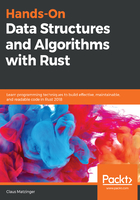
Unsafe
Rust's code is "safe" because the compiler checks and enforces certain behavior when it comes to memory access and management. However, sometimes these rules have to be forgone, making the code unsafe. unsafe is a keyword in Rust and declares a section of code that can do most of the things the C programming language would let you do. For example, it lets the user do the following (from the Rust Book, chapter 19.1):
- Dereference a raw pointer
- Call an unsafe function or method
- Access or modify a mutable static variable
- Implement an unsafe trait
These four abilities can be used for things such as very low-level device access, language interoperability (the compiler can't know what native libraries do with their memory), and so on. In most cases, and certainly in this book, unsafe is not required. In fact, the Rustonomicon (https://doc.rust-lang.org/nomicon/what-unsafe-does.html) defines a list of issues the language is trying to prevent from happening by providing the safe part:
- Dereferencing null, dangling, or unaligned pointers.
- Reading uninitialized memory.
- Breaking the pointer aliasing rules.
- Producing invalid primitive values:
- Dangling/null references
- Null fn pointers
- A bool that isn't 0 or 1
- An undefined enum discriminant
- A char outside the ranges [0x0, 0xD7FF] and [0xE000, 0x10FFFF]
- A non-UTF8 string
- Unwinding into another language.
- Causing a data race.
The fact that these potential issues are prevented in safe Rust certainly makes the life of a developer easier, especially when designing algorithms or data structures. As a consequence, this book will always work with safe Rust.