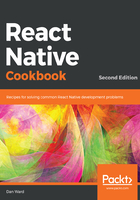
上QQ阅读APP看书,第一时间看更新
How to do it...
- The first thing we're going to do is create a new folder called Images in the root of the project. Add the images you've downloaded to the new folder.
- In the App.js file, we include all of the dependencies we'll need for this component:
import React from 'react'; import { StyleSheet, View, Image } from 'react-native';
- We need to require the images that'll be displayed in our component. By defining them in constants, we can use the same image in different places:
const playIcon = require('./images/play.png');
const volumeIcon = require('./images/sound.png');
const hdIcon = require('./images/hd-sign.png');
const fullScreenIcon = require('./images/full-screen.png');
const flower = require('./images/flower.jpg');
const remoteImage = { uri: `https://farm5.staticflickr.com/4702/24825836327_bb2e0fc39b_b.jpg` };
- We're going to use a stateless component to render the JSX. We'll use all of the images we've declared in the previous step:
export default class App extends React.Component { render() { return ( <View style={styles.appContainer}> <ImageBackground source={remoteImage} style=
{styles.videoContainer} resizeMode="contain"> <View style={styles.controlsContainer}> <Image source={volumeIcon} style={styles.icon} /> <View style={styles.progress}> <View style={styles.progressBar} /> </View> <Image source={hdIcon} style={styles.icon} /> <Image source={fullScreenIcon} style={styles.icon} /> </View> </ImageBackground> </View> ); } };
- Once we have the elements that we're going to render, we need to define the styles for each element:
const styles = StyleSheet.create({ flower: { flex: 1, }, appContainer: { flex: 1, justifyContent: 'center', alignItems: 'center', }, videoContainer: { backgroundColor: '#000', flexDirection: 'row', flex: 1, justifyContent: 'center', alignItems: 'center', }, controlsContainer: { padding: 10, backgroundColor: '#202020', flexDirection: 'row', alignItems: 'center', marginTop: 175, }, icon: { tintColor: '#fff', height: 16, width: 16, marginLeft: 5, marginRight: 5, }, progress: { backgroundColor: '#000', borderRadius: 7, flex: 1, height: 14, margin: 4, }, progressBar: { backgroundColor: '#bf161c', borderRadius: 5, height: 10, margin: 2, paddingTop: 3, width: 80, alignItems: 'center', flexDirection: 'row', }, });
- We're done! Now, when you view the application, you should see something like the following:
