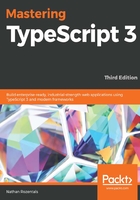
Function return types
Using the very simple syntactic sugar that TypeScript uses, we can easily define the type of a variable that a function should return. In other words, when we call a function, and it returns a value, what type should the return value be treated as?
Consider the following TypeScript code:
function addNumbers(a: number, b: number) : string { return a + b; } var addResult = addNumbers(2,3); console.log(`addNumbers returned : ${addResult}`);
Here, we have added a :number type to both of the parameters of the addNumbers function (a and b), and we have also added a :string type just after the ( ) braces in the function definition. Placing a type annotation after the function definition means that we are defining the return type of the entire function. In our example, then, the return type of the addNumbers function must be of type string.
Unfortunately, this code will generate an error message as follows:
error TS2322: Type 'number' is not assignable to type 'string'
This error message is indicating that the return type of the addNumbers function must be a string. Taking a closer look at the code, we will note that the offending code is, in fact, return a + b. Since a and b are numbers, we are returning the result of adding two numbers, which is of type number. To fix this code, then, we need to ensure that the function returns a string, as follows:
function addNumbers(a: number, b: number) : string { return (a + b).toString(); }
This code will now compile correctly, and will output the following:
addNumbers returned : 5