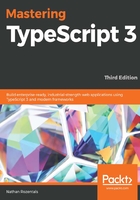
Generic syntax
As an example of TypeScript generic syntax, let's write a class called Concatenator that will concatenate the values in an array. We will need to ensure that each element of the array is of the same type. This class should be able to handle arrays of strings, arrays of numbers, and, in fact, arrays of any type. In order to do this, we need to rely on functionality that is common to each of these types. As all JavaScript objects have a toString function (which is called whenever a string is needed by the runtime), we can use this toString function to create a generic class that outputs all values held within an array.
A generic implementation of this Concatenator class is as follows:
class Concatenator< T > { concatenateArray(inputArray: Array< T >): string { let returnString = ""; for (let i = 0; i < inputArray.length; i++) { if (i > 0) returnString += ","; returnString += inputArray[i].toString(); } return returnString; } }
The first thing we notice is the syntax of the class declaration, Concatenator < T >. This < T > syntax is the syntax used to indicate a generic type, and the name used for this generic type in the rest of our code is T. The concatenateArray function also uses this generic type syntax, Array < T >. This indicates that the inputArray argument must be an array of the type that was originally used to construct an instance of this class.