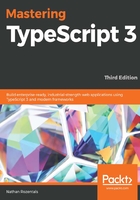
Parameter decorators
The final type of decorator that we will cover are parameter decorators. Parameter decorators are used to decorate the parameters of a particular method. As an example, consider the following code:
function parameterDec(
target: any, methodName : string, parameterIndex: number)
{ console.log(`target: ${target}`); console.log(`methodName : ${methodName}`); console.log(`parameterIndex : ${parameterIndex}`); }
Here, we have defined a function named parameterDec, with three arguments. The target argument will contain the class prototype, as we have seen before. The methodName argument will contain the name of the method that contains the parameter, and the parameterIndex argument will contain the index of the parameter. We can use this parameter decorator function, as follows:
class ClassWithParamDec { print(@parameterDec value: string) { } }
Here, we have a class named ClassWithParamDec, that contains a single print function. This print function has a single argument named value, which is of type string. We have decorated this value parameter with the parameterDec decorator. Note that the syntax for using a parameter decorator (@parameterDec) is the same as any other decorator. The output of this code is as follows:
target: [object Object] methodName : print parameterIndex : 0
Note that we are not given any information about the parameter that we are decorating. We are not told what type it is, or even what its name is. Parameter decorators, therefore, can only really be used to establish that a parameter has been declared on a method.