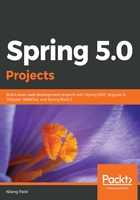
Subscriber rules
Subscriber has the following interface definition:
public interface Subscriber<T> {
public void onSubscribe(Subscription s);
public void onNext(T t);
public void onError(Throwable t);
public void onComplete();
}
Rule number 2.1 says, A Subscriber must signal demand via Subscription.request(long n) to receive onNext signals. This rule is in line with Publisher rule number 1.1 in the sense that it establishes the responsibility of Subscriber to inform when and how many messages it is able and willing to receive.
Rule number 2.4 says, .onComplete() and Subscriber.onError(Throwable t) must consider the Subscription cancelled after having received the signal. Here again, the design intention at play is highlighted. The design sequence of sending ensures that the process of the message being sent from Publisher to Subscriber is completely decoupled. Therefore, Publisher is not bound by the Subscriber intent to keep listening, hence ensuring a non-blocking arrangement.
As soon as Publisher sends out a message, it has no messages to be sent with Subscriber.onComplete() and the Subscription object is no longer valid/available. This is similar to when an exception is thrown back with Subscriber.onError(Throwable t). The Subscription object can no longer be utilized by Subscriber to request more messages.
It is worthwhile mentioning another couple of rules around the same design. These are rules number 2.9 and 2.10 concerning Subscription.request(long n). The rule says that a Subscriber can get the onError signal or the onComplete signal with or without a preceding call to Subscription.request(long n).