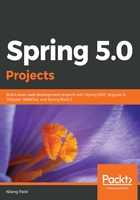
Basics of Reactive Programming
In a procedural programming model, a task is described as a series of actions executed in a sequential order. On the other hand, the Reactive Programming model facilitates the necessary arrangement to propagate the changes, which help in deciding what to do instead of how to do it.
Let's understand the concept with a very basic example, as follows:
int num1=3;
int num2=5;
int num3 = num1 + num2;
System.out.println("Sum is -->"+num3);
num1=6;
num2=8;
System.out.println("Sum is -->"+num3);
This is what we generally do in a procedural programming style. In this code, we are simply doing a summation of two numbers assigned to the third number and then printing it. In the next line, we are changing the value of the initial two numbers, but it doesn't update the third number. This is because num1 + num2 is evaluated and assigned to num3 on that line only. Now consider the same equation in an Excel sheet as follows:

In this case, the changes of the E and F columns always listen to the G column. This is what Reactive Programming does. It propagates the changes to the one interested in those changes.
You might have used Reactive Programming unknowingly in your day-to-day coding practice. For example, if you have created a user registration screen where you validate the username entered by a user who is already present in the system, makes an Ajax call and shows an appropriate message saying This username is already used.
Another example is the listener or callback function that you define with a mouse click or keystroke on the web page. In these cases, mouse click and focus out events (for username validation) can be considered as a stream of events that you can listen to and execute appropriate action or functions on.
This is just one usage of the event stream. Reactive programming allows you to observe and react to any changes caused by the stream of events, like changes in a database, user input, property updates, data from external resources, and so on. Let's understand it by taking a real-life example.
Let's say you want to invest in mutual funds and there are many companies who provide facilities to invest on your behalf. They also produce statistics about the performance of various funds along with their history, market share, capital investment ratio, and so on. Based on this, they give some categories like moderate risk, low risk, moderately high risk, high risk, and so on. They also give a rating based on the performance of each fund.
The rating and the category will suggest that users choose a particular fund based on their requirement (short term, long term, and so on) and the type of risk they can afford. The changes happen in the rating and the category can be considered as an event (or data) stream that will cause a system to change the suggestion to the user. Another practical example of a data stream would be a social media feed, such as Facebook, Twitter, and so on.
Function reactive is a paradigm of reacting to the data stream in a functional way, providing additional features such as filters, combine, buffers, maps, and lot others. Using them, you can perform certain operations on a data stream, which help it to react in a better way. Taking the previous example of a mutual fund, the filter function can be used to suggest only those funds that are safe for investment in a real-time manner.
Reactive Programming is mainly used to build an interactive user interface and other systems that need time interaction, such as graphical applications, animations, simulations, chatbots, and so on.