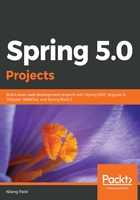
上QQ阅读APP看书,第一时间看更新
Using Hibernate Validator to add validations
There are a few checks we need to add to our model classes so that the data being sent from the UI is not invalid. For this, we will make use of Hibernate Validator. You are required to add the Hibernate dependency as follows:
<properties>
<java.version>1.8</java.version>
<lombok.version>1.16.18</lombok.version>
<hibernate.validator.version>6.0.2.Final</hibernate.validator.version>
</properties>
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>${hibernate.validator.version}</version>
</dependency>
Now go back to com.nilangpatel.worldgdp.model.Country and update it with the following:
@Data public class Country {
@NotNull @Size(max = 3, min = 3) private String code;
@NotNull @Size(max = 52) private String name;
@NotNull private String continent;
@NotNull @Size(max = 26) private String region;
@NotNull private Double surfaceArea;
private Short indepYear;
@NotNull private Long population;
private Double lifeExpectancy;
private Double gnp;
@NotNull private String localName;
@NotNull private String governmentForm;
private String headOfState;
private City capital;
@NotNull private String code2;
}
Next is to update the com.nilangpatel.worldgdp.model.City class in a similar way, as follows:
@Data public class City {
@NotNull private Long id;
@NotNull @Size(max = 35) private String name;
@NotNull @Size(max = 3, min = 3) private String countryCode;
private Country country;
@NotNull @Size(max = 20) private String district;
@NotNull private Long population;
}
And finally, update com.nilangpatel.worldgdp.model.CountryLanguage class as well, as follows:
@Data
public class CountryLanguage {
private Country country;
@NotNull private String countryCode;
@NotNull @Size(max = 30) private String language;
@NotNull @Size(max = 1, min = 1) private String isOfficial;
@NotNull private Double percentage;
}