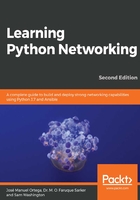
上QQ阅读APP看书,第一时间看更新
Accessing Twitter API resources
The created object has access to the GET and POST resources of the Twitter API, which are listed in the reference index: https://developer.twitter.com/en/docs/api-reference-index.
The Twitter package transforms the responses of the Twitter API, of JSON format, to a Python object. The Twitter API uses the JSON format by default to transmit information, but the Twitter module transforms the information into a dictionary-type object. This query returns the last two tweets related to Python in the English language:
>>> search= twitter.search.tweets(q="python", lang="en", count="2")
>>> for item in search.keys():
>>> print(item)
In this script, we can see an example of an application that consumes the Twitter API, gets a search term from the user input, and saves the results in a JSON file. You can find the following code in the twitter_search.py file:
#! /usr/bin/python3
import twitter, json
def twitter_connection(file):
'''Create the object from which the Twitter API will be consumed,reading the credentials from a file, defined in path parameter.'''
(CONSUMER_KEY,CONSUMER_SECRET,OAUTH_TOKEN,OAUTH_TOKEN_SECRET) = open(file, 'r').read().splitlines()
auth = twitter.oauth.OAuth(OAUTH_TOKEN,OAUTH_TOKEN_SECRET,CONSUMER_KEY,CONSUMER_SECRET)
return twitter.Twitter(auth=auth)
def recently_tweets(tw, search_term):
'''Get the last 10 tweets in English from a specific search.'''
search = tw.search.tweets(q=search_term, lang="en", count="10")["statuses"]
print(search)
return search
def save_tweets(tweets, file):
'''Store the tweets in JSON format in the specified file.'''
with open(file, "w") as f:
json.dump(tweets, f, indent=1)
def main(file='tweets.json'):
try:
search_term = input("Enter the search term in twitter : ")
tw = twitter_connection("credentials.txt")
tweets = recently_tweets(tw, search_term)
save_tweets(tweets, file)
except Exception as e:
print(str(e))
if __name__ == "__main__":
main()