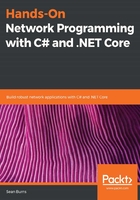
The System.Net.UriBuilder class
If you've made it all the way through this elaborate definition of the URL specification, you might be wondering how on earth you can leverage this in your code to access a resource, when you already know specifically where to look for it. Enter, dear readers, the UriBuilder class!
Living in the System.Net namespace, the UriBuilder class is a factory class for generating instances of the Uri class. It provides users with several overloaded constructors to allow the specification of more of the components of a valid URL progressively. It also provides accessors to properties representing each of those components individually. Finally, it provides a function to produce the well-formed instance of the Uri class from the component parts.
Let's start with a very simple example. We'll use UriBuilder to compose an instance of Uri with only the Scheme and Host components as follows:
public Uri GetSimpleUri() {
var builder = new UriBuilder();
builder.Scheme = "http";
builder.Host = "packt.com";
return builder.Uri;
}
With this method, we can see how the UriBuilder class composes a well-formed and syntactically correct Uri out of the component parts we specify, as demonstrated in the following code snippet:
using System;
using System.Net;
using System.Threading;
namespace UriTests {
public class TestUriProgram {
public static Uri GetSimpleUri() {
//...
}
public static void Main(string[[ args) {
var simpleUri = GetSimpleUri();
Console.Warn(simpleUri.ToString());
Thread.Sleep(10000);
}
}
}
By running this program, you should see the http://packt.com output while your console is open for ten seconds, before it closes and the application terminates.
Here, we didn't need to specify that the http component of the URL should be followed by a colon character. We didn't say anything about the host we specified being prefixed with the // prefix characters. The UriBuilder class did that for us. This factory class gives us a clean way to incrementally construct a more specific desired location, without us, as the developers, having to keep the nitty-gritty details of delimiters, prefixes, and suffixes in our heads all the time.
In this example, we leveraged the fact that the UriBuilder class provides public get access to all of the properties that it has to encapsulate each component of a Uri. However, you can also apply many of those properties through a series of overloaded constructors, if you know their values at the time of construction.
The UriBuilder class has seven overloaded constructors. We've seen the default constructor, taking no parameters, but now let's look at a program that leverages each of the constructors and see what they provide. Given that we know the transport scheme and domain name we intend to look up, we can simplify our initial method for a simple Uri as follows:
public static Uri GetSimpleUri_Constructor() {
var builder = new UriBuilder("http", "packt.com");
return builder.Uri;
}
With that change, the output from our TestUriProgram will print the exact same string we saw before, but the code to produce that output is one-third of the size. Whenever possible, I recommend using the constructor overloads to instantiate the UriBuilder class. Doing so shrinks our code height and makes our intentions explicit when instantiating the class. Always be more explicit in your code when possible.