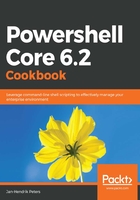
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- The Get-Member cmdlet is again an indispensable tool when working with .NET classes. Try the following bit of code to list all object methods of the File and Directory objects:
Get-ChildItem | Get-Member -MemberType Methods
- Execute the following commands:
# Accessing methods
$file = New-TemporaryFile
$parentPath = Split-Path -Path $file.FullName -Parent
$newPath = Join-Path -Path $parentPath -ChildPath newFileName
# With arguments
$file.MoveTo($newPath)
$file.FullName # The MoveTo method is a way of changing the FullName property, which is read-only.
# and without arguments
$file.Delete()
- Be careful when accessing methods that do not exist, or methods of objects that do not exist. Try to do so now by calling a method that does not exist:
# Careful with empty objects or non-existing methods
# Method does not exist. Throws a terminating MethodNotFound error
$file.DoStuff()
# Object does not exist. Throws an InvokeMethodOnNull exception.
($null).Delete()
- Execute the following code:
# Finding possible arguments
# CopyTo has two overloads
($file | Get-Member -Name CopyTo).Definition
# A handy shortcut is simply leaving the parentheses off
$file.CopyTo
- Now execute the following code:
# Performance of .NET calls versus cmdlets
Measure-Command -Expression {
Get-ChildItem -Recurse -File -Path /
}
Measure-Command -Expression {
$root = Get-Item -Path /
$options = [System.IO.EnumerationOptions]::new()
$options.RecurseSubdirectories = $true
$root.GetFiles('*', $options)
}
- Methods may or may not return objects. If they do, Get-Member will display the data type that is returned for each overload:
# Method calls may or may not return values
$process = Get-Process -Id $PID
$process | Get-Member -Name WaitForExit
# The WaitForExit method has two overloads
# When passing a timeout to WaitForExit, it returns a boolean value indicating if the process
# has exited in the allotted timeout.
$pingProcess = if ($IsWindows)
{
Start-Process -FilePath ping -ArgumentList 'microsoft.com','-n 10' -PassThru
}
else
{
Start-Process -FilePath ping -ArgumentList 'microsoft.com','-n 10' -PassThru
}
# Waiting 500ms should return false, since our 10 ICMP request will most likely not be done
if( -not $pingProcess.WaitForExit(500))
{
Write-Host -ForegroundColor Yellow "Pinging not yet complete..."
}
# The other overload has no return type (void) and will wait indefinitely
$pingProcess.WaitForExit()