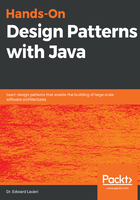
Method call chaining
OOP affords us the opportunity to use method call chaining. That is the process of making multiple method calls in a single statement. Here is the syntax:
object.method1().method2().method3().method4();
Using the syntax provided previously, we can walk through the process of method call chaining. The object first calls method1(), which returns the calling object. Next, that returned object calls method2(), which returns the calling object. You can see the process here. It works from left to right.
So, we can implement this in our Driver class, as shown here:
// Example using method call chaining
Bicycle myBike5 = new Bicycle(24, 418.50, 17.2, "Green");
myBike5.setColor("Peach").setGears(32).outputData("Number 5");
Before this code can work, we will need to make two changes to each method we want to use in our method call chaining:
- Add a return type to the method definition
- Add a return this; statement to each method
Here is an example of the setGears() method that the preceding changes listed made:
public Bicycle setGears(int nbr) {
this.gears = nbr;
return this;
}
Once these changes are made, we can run the Driver class:

The preceding screenshot shows the final result.