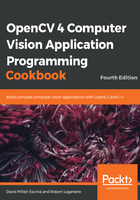
How it works...
All binary arithmetic functions work the same way. Two inputs are provided and a third parameter specifies the output. In some cases, weights that are used as scalar multipliers in the operation can be specified. Each of these functions comes in several flavors; cv::add is a good example of a function that is available in many forms:
// c[i]= a[i]+b[i]; cv::add(imageA,imageB,resultC); // c[i]= a[i]+k; cv::add(imageA,cv::Scalar(k),resultC); // c[i]= k1*a[1]+k2*b[i]+k3; cv::addWeighted(imageA,k1,imageB,k2,k3,resultC); // c[i]= k*a[1]+b[i]; cv::scaleAdd(imageA,k,imageB,resultC);
For some functions, you can also specify a mask:
// if (mask[i]) c[i]= a[i]+b[i]; cv::add(imageA,imageB,resultC,mask);
If you apply a mask, the operation is performed only on pixels for which the mask value is not null (the mask must be one-channel). Have a look at the different forms of the cv::subtract, cv::absdiff, cv::multiply, and cv::divide functions. Bitwise operators (operators applied to each individual bit of the pixels' binary representations) are also available: cv::bitwise_and, cv::bitwise_or, cv::bitwise_xor, and cv::bitwise_not. The cv::min and cv::max operators, which find the per-element maximum or minimum pixel values, are also very useful.
In all cases, the cv::saturate_cast function (see the preceding recipe) is always used to make sure that the results stay within the defined pixel value domain (that is, to avoid overflow or underflow).
The images must have the same size and type (the output image will be reallocated if it does not match the input size). Also, since the operation is performed per element, one of the input images can be used as the output.
Several operators that take a single image as the input are also available—cv::sqrt, cv::pow, cv::abs, cv::cuberoot, cv::exp, and cv::log. In fact, there exists an OpenCV function for almost any operation you have to apply on image pixels.