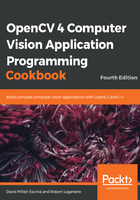
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's write the following test program that will allow us to test the different properties of the cv::Mat data structure, as follows:
- Include the opencv headers and a c++ i/o stream utility:
#include <iostream> #include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui.hpp>
- We are going to create a function that generates a new gray image with a default value for all its pixels:
cv::Mat function() { // create image cv::Mat ima(500,500,CV_8U,50); // return it return ima; }
- In the main function, we are going to create six windows to show our results:
// define image windows cv::namedWindow("Image 1"); cv::namedWindow("Image 2"); cv::namedWindow("Image 3"); cv::namedWindow("Image 4"); cv::namedWindow("Image 5"); cv::namedWindow("Image");
- Now, we can start to create different mats (with different sizes, channels, and default values) and wait for the key to be pressed:
// create a new image made of 240 rows and 320 columns cv::Mat image1(240,320,CV_8U,100); cv::imshow("Image", image1); // show the image cv::waitKey(0); // wait for a key pressed // re-allocate a new image image1.create(200,200,CV_8U); image1= 200; cv::imshow("Image", image1); // show the image cv::waitKey(0); // wait for a key pressed // create a red color image // channel order is BGR cv::Mat image2(240,320,CV_8UC3,cv::Scalar(0,0,255)); // or: // cv::Mat image2(cv::Size(320,240),CV_8UC3); // image2= cv::Scalar(0,0,255); cv::imshow("Image", image2); // show the image cv::waitKey(0); // wait for a key pressed
- We are going to read an image with the imread function and copy it to another mat:
// read an image cv::Mat image3= cv::imread("puppy.bmp"); // all these images point to the same data block cv::Mat image4(image3); image1= image3; // these images are new copies of the source image image3.copyTo(image2); cv::Mat image5= image3.clone();
- Now, we are going to apply an image transformation (flip) to a copied image, show all images created, and wait for a keypress:
// transform the image for testing cv::flip(image3,image3,1); // check which images have been affected by the processing cv::imshow("Image 3", image3); cv::imshow("Image 1", image1); cv::imshow("Image 2", image2); cv::imshow("Image 4", image4); cv::imshow("Image 5", image5); cv::waitKey(0); // wait for a key pressed
- Now, we are going to use the function created before to generate a new gray mat:
// get a gray-level image from a function cv::Mat gray= function();
cv::imshow("Image", gray); // show the image cv::waitKey(0); // wait for a key pressed
- Finally, we are going to load a color image but convert it to gray in the loading process. Then, we will convert its values to float mat:
// read the image in gray scale image1= cv::imread("puppy.bmp", IMREAD_GRAYSCALE); image1.convertTo(image2,CV_32F,1/255.0,0.0); cv::imshow("Image", image2); // show the image cv::waitKey(0); // wait for a key pressed
Run this program and take a look at the following images produced:

Now, let's go behind the scenes to understand the code better.