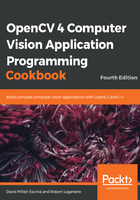
Drawing on images
OpenCV also offers a few functions to draw shapes and write texts on images. The examples of basic shape-drawing functions are circle, ellipse, line, and rectangle. The following is an example of how to use the circle function:
cv::circle(image, // destination image cv::Point(155,110), // center coordinate 65, // radius 0, // color (here black) 3); // thickness
The cv::Point structure is often used in OpenCV methods and functions to specify a pixel coordinate. Note that here we assume that the drawing is done on a gray-level image; this is why the color is specified with a single integer. In the next recipe, you will learn how to specify a color value in the case of color images that use the cv::Scalar structure. It is also possible to write text on an image. This can be done as follows:
cv::putText(image, // destination image "This is a dog.",// text cv::Point(40,200), // text position cv::FONT_HERSHEY_PLAIN, // font type 2.0, // font scale 255, // text color (here white) 2); // text thickness
Calling these two functions on our test image will then result in the following screenshot:

Let's see what happens when you run the example using Qt.