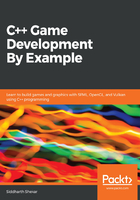
Variables
A variable is used to store a value in it. Whatever value you store in a variable is stored in the memory location associated with that memory location. You assign a value to a variable with the following syntax.
We can first declare a variable type by specifying a type and then the variable name:
Type variable;
Here, type is the variable type and variable is the name of the variable.
Next, we can assign a value to a variable, which is done like so:
Variable = value;
Now that value is assigned to the variable.
Or, you can both declare the variable and assign a value to it in a single line, as follows:
type variable = value;
Before you set a variable, you have to specify the variable type. You can then use the equal to (=) sign to assign the value to the variable.
Let's look at some example code:
#include <iostream>
#include <conio.h>
// Program prints out value of n to screen int main() { int n = 42; std::cout <<"Value of n is: "<< n << std::endl;
_getch();
return 0; }
Replace the previous code with this code in Source.cpp and run the application. This is the output you should get:

In this program, we specify the data type as int. An int is a C++ data type that can store integers. So, it cannot store decimal values. We declare a variable called n and then we assign a value of 42 to it. Do not forget to add the semicolon at the end of the line.
In the next line, we print the value to the console. Note that, to print the value of n, we just pass in n in cout and don't have to add quotation marks.
On a 32-bit system, an int variable uses 4 bytes (which is equal to 32 bits) of memory. This basically means the int data type can hold values between 0 and 232-1 (4,294,967,295). However, one bit is needed to describe the sign for the value (positive or negative), which leaves 31 bits remaining to express the actual value. Therefore, a signed int can hold values between -231 (-2,147,483,648) and 231-1 (2,147,483,647).
Let's look at some other data types:

- bool: A bool can have only two values. It can either store true or false.
- char: This stores integers ranging between -128 and 127. char or character variables are used to store ASCII characters such as single characters—letters, for example.
- short and long: These are also integer types but these are able to store more information than just int. The size of int is system-dependent and long and short have fixed sizes irrespective of the system used.
- float: This is a floating point type. This means that it can store values with decimal spaces such as 3.14, 0.000875 and -9.875. It can store data with up to seven decimal places.
- double: This is a float with more precision. It can store decimal values up to 15 decimal places.
You also have unsigned data types of the same data type used to maximize the range of values it can store. Unsigned data types are used to store positive values. Consequently,all unsigned values start at 0.
So, char and unsigned char can store positive values from 0 to 255. Similar to unsigned char we have unsigned short, int, and long.
You can assign values to bool, char, and float as follows:
#include <iostream> #include <conio.h> // Program prints out value of bool, char and float to screen int main() { bool a = false; char b = 'b'; float c = 3.1416f; unsigned int d = -82; std::cout << "Value of a is : " << a << std::endl; std::cout << "Value of b is : " << b << std::endl; std::cout << "Value of c is : " << c << std::endl; std::cout << "Value of d is : " << d << std::endl; _getch(); return 0; }
This is the output when you run the application:

Everything is printing fine except d, which was assigned -82. What happened here? Well that's because d can store only unsigned values, so if we assign it -82, it gives a garbage value. Change it to just 82 without the negative sign and it will print the correct value:

Unlike int, bool stores a binary value where false is 0 and true is 1. So, when you print out the values of true and false, the output will be 1 and 0.
char store characters specified with single quotation marks, and values with decimals are printed just how you stored the values in the floats. The f is added at the end of the value when assigning a float, to tell the system that it is a float and not a double.