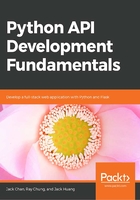
Configuring Endpoints
Now that we have defined all our resources, we will set up some endpoints so that users can send requests to them. These endpoints can be accessed by the users and are connected to specific resources. We will be using the add_resource method on the API object to specify the URL for these endpoints and route the client HTTP request to our resources.
For example, the api.add_resource(RecipeListResource, '/recipes') syntax is used to link the route (relative URL path) to RecipeListResource so that HTTP requests will be directed to this resource. Depending on the HTTP verb (for example, GET, and POST), the request will be handled by the corresponding methods in the resource accordingly.
Exercise 10: Creating the Main Application File
In this exercise, we will create our app.py file, which will be our main application file. We will set up Flask and initialize our flask_restful.API there. Finally, we will set up the endpoints so that users can send requests to our backend services. Let's get started:
- Create the app.py file under the project folder.
- Import the necessary classes using the following code:
from flask import Flask
from flask_restful import Api
from resources.recipe import RecipeListResource, RecipeResource, RecipePublishResource
- Set up Flask and initialize flask_restful.API with our Flask app:
app = Flask(__name__)
api = Api(app)
- Add resource routing by passing in the URL so that it will route to our resources. Each resource will have its own HTTP method defined:
api.add_resource(RecipeListResource, '/recipes')
api.add_resource(RecipeResource, '/recipes/<int:recipe_id>')
api.add_resource(RecipePublishResource, '/recipes/<int:recipe_id>/publish')
if __name__ == '__main__':
app.run(port=5000, debug=True)
Note
In RecipeListResource, we have defined the get and post methods. So, when there is a GET HTTP request to the "/recipes" URL route, it will invoke the get method under RecipeListResource and get back all the published recipes.
In the preceding code, you will notice that we have used <int: recipe_id > in the code. It is there as a placeholder for the recipe ID. When a GET HTTP request has been sent to the route "/recipes/2" URL, this will invoke the get method under RecipeResource with a parameter, that is, recipe_id = 2.
- Save app.py and right-click on it to run the application. Flask will then start up and run on the localhost (127.0.0.1) at port 5000:

Figure 2.9: Flask started and running on localhost
Congratulations! You have completed the API endpoint. Now, let's move on to testing. You can either test it in curl/httpie or Postman.