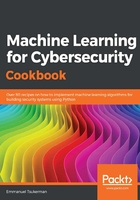
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's see how to generate text using Markov chains by performing the following steps:
- Start by importing the markovify library and a text file whose style we would like to imitate:
import markovify
import pandas as pd
df = pd.read_csv("airport_reviews.csv")
As an illustration, I have chosen a collection of airport reviews as my text:
"The airport is certainly tiny! ..."
- Next, join the individual reviews into one large text string and build a Markov chain model using the airport review text:
from itertools import chain
N = 100
review_subset = df["content"][0:N]
text = "".join(chain.from_iterable(review_subset))
markov_chain_model = markovify.Text(text)
Behind the scenes, the library computes the transition word probabilities from the text.
- Generate five sentences using the Markov chain model:
for i in range(5):
print(markov_chain_model.make_sentence())
- Since we are using airport reviews, we will have the following as the output after executing the previous code:
Surprisingly realistic! Although the reviews would have to be filtered down to the best ones.
- Generate 3 sentences with a length of no more than 140 characters:
for i in range(3):
print(markov_chain_model.make_short_sentence(140))
With our running example, we will see the following output: