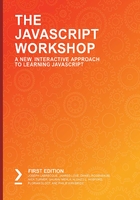
Framework versus Library
Library describes an external collection of functions that perform a given task. These functions are made accessible to us as users of the library via APIs. One useful library is lodash, which can, for example, remove all duplicated values from an array:
const duplicatedArray = [1,2,1,2,3];
const uniqueArray = lodash.uniq(duplicatedArray)
// => [1,2,3]
Frameworks, on the other hand, are a particular form of library. They are reusable code frames that build the foundation of a JavaScript application. In contrast to libraries, which extend your code with functionality, a framework can stand alone and is enhanced with your source code to create an app as you like.
A popular framework is Vue.js, which we can use as follows:
library-vue.js
1 // example.html
2 <div id="example">
3 <input :value="text" @input="update"/>
4 <div v-html="myOwnText"></div>
5 </div>
6 //————————————————————————————————
7 // example.js
8 new Vue({
9 el: '#example',
10 data: {
11 text: 'My first framework'
12 },
13 computed: {
14 myOwnText: function () {
15 return this.text
16 }
The full code is available at: https://packt.live/32MD4IN
As you can see, in general, there is more complexity to a framework than there is to a library. Nonetheless, both are equally important to software development.
Despite the technical differences between libraries and frameworks, we are going to use those terms interchangeably. Another synonym you'll encounter in the JavaScript world to describe external source code is "package." One of those packages you may encounter in JS resources is Vanilla.js. We'll have a look at it in the next section.
Vanilla.js
This specific framework follows the informal convention of including the JavaScript file extension with the name nameOfFramework.js. However, vanilla.js is not a framework; it's not even a library. People referring to vanilla.js are talking about plain JavaScript without any external code or tooling. The name is a running gag within the JavaScript community because some developers and non-developers think we need to use a framework for everything we build. We will discuss why this isn't the case later.