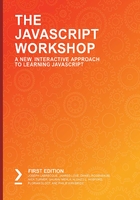
Data Types
Programming is all about manipulating data. Data can represent values such as people's names, temperature, image dimensions, the amount of disk storage, and total likes on a discussion group post.
All the data in a program has a data type. The data types that you usually learn to use first in JavaScript are number, string, boolean, object, array, and function. The number, string, and Boolean data types represent a single value. Objects represent more complex data. Functions are for writing programs.
Some common JavaScript data types with their uses and descriptions are as follows:
- number: Any positive or negative value whole numbers, usually called integers and floating-point numbers, that can be used in mathematical operations. It is used in product prices, checkout totals, the number of likes on a post, the geometry value of Pi, and can be used as a random number.
- string: Any set of valid characters that cannot be, or are not intended to be, used in computational operations. They are used to comment on a discussion post which can be a company name, a street address, name of a place, an account number, a telephone number, or a postal number.
- boolean: Any value representing true and false. It is used to check whether a form can be submitted, whether a password meets its required characters, whether an order balance qualifies for free shipping, and whether a button can be clicked.
- object: An unordered collection of values, called properties, and code, called methods, that are intended to work together. It is used for real-world objects such as an order, stopwatch, clock, date, or microwave. They can be used for software objects such as a web page document, an HTML element on a web page, a CSS style rule, or an HTTP request.
- function: A specialized object data type that represents a block of code. The code can use optional input data and optionally return data. They can be used for the conversion of data such as temperature, finding a value in a list, updating the style of an HTML element, sending data to a web server, revealing a message on the screen, or checking for valid data entry formats such as an email address.
Representation of Data
Data is represented in programs using expressions. If you've ever worked with a spreadsheet program, then expressions are analogous to cell formulas. Expressions can be resolved to a value representing a specific data type.
Expressions can be broken down into smaller parts, as follows:
- Literal values
- Operators
- Variables
- Functions that return data
- Object properties
- Object methods that return data
A good place to start learning about expressions is with literal values, operators, and variables. Functions and objects are covered separately, later in this chapter, and we will revisit them through their use in expressions.
Literal Values
Literal values are written into the programming code. Literal values are static. This means that they have the same value every time the line of code is executed, and they cannot be changed.
Literal data needs to be formatted according to the rules for its data type. Number, string, and Boolean values are a good place to start so that we can understand the formatting of literal values. Functions and object literals are covered in their own topics later. Here is a list of some of their types and their rules and an example of each valid and invalid case:
- Number: Numbers can appear in expressions. They cannot include formatting punctuation, such as currency symbols, comma separators, and spaces. Negative numbers are preceded with the minus symbol. Some valid examples include 1000000, 101, 9.876, and -0.1234. Invalid examples include 1,000,000, $1000000, and 1 000 000.
- String: String data is encased in delimiters. The delimiters are either single or double quotes. Single quotes can appear within a double-quote delimited string, and double quotes can appear within a single-quote delimited string. Also, the escape character, \, is a delimiter that needs to appear as part of a string; for example, "ABC Company". Valid examples include 'ABC Company', "Earth's Moon", "She yelled \"duck\"!", 'She yelled "duck"!', and so on. Invalid examples include ABC Company, and "She yelled "duck"!".
- Boolean: There are two keywords: true and false. They are lowercase. true and false are valid examples, whereas invalid examples include True, TRUE, FALSE, and False.
Using Operators in Expressions
Operators are used to performing arithmetic, combine text, make logical comparisons, and assign values to variables and properties.
The operators we look at can be grouped as follows:
- Arithmetic
- String
- Grouping
- Comparison
- Logical
- typeof
For math computations, we use arithmetic operators. The string operator allows us to combine parts of an expression into a string value. The following table describes certain arithmetic operators and some examples:

Figure 3.1: Arithmetic operators
Let's say we could use +, which is concatenation. It converts non-string data types into strings. The following code shows three separate examples:
"Blue" + "Moon"
"Blue" + " " + "Moon"
"$" + 100 * .10 + " discount"
The output for each would be as follows:
"BlueMoon"
"Blue Moon"
"$10 discount"
Expressions are not evaluated left to right. Instead, they are evaluated based on a preset operator order, which is called the operator precedence. For example, the multiplication operator has higher precedence than the addition operator does. You can override the operator's precedence using the grouping operator. It forces the evaluation of the expression contained within it before the rest of the expression is evaluated.
For example, the () operator controls the precedence of expression evaluation:
1 + 2 * 3
(1 + 2) * 3
10 + 10 * 5 + 5
(10 + 10) * (5 + 5)
The output for each of the preceding examples would be as follows:
7
9
65
100
Comparing data is an important part of programming. The resulting value of comparing data is either true or false. A portion of an expression can be compared to another portion using the comparison operators, which are sometimes called relational operators. The following table describes certain comparison operators, along with examples:

Figure 3.2: Comparison operators
Multiple parts of an expression can be compared using logical operators. These are sometimes called Boolean operators. Some Boolean operators, along with a description of them and examples, are as follows:

Figure 3.3: Logical operators
Not all operators are symbols. A very helpful operator is typeof. It shows the data type as a string. The operator is all lowercase letters. Use the group operator to get the type of an expression using other operators.
For example, the typeof operator controls the precedence of expression evaluation:
typeof 100
TypeOf 100
typeof "100"
typeof true
typeof (1 > 2)
typeof (2 + " dozen eggs")
The output for each of the preceding examples would be as follows:
number
Uncaught SyntaxError: …
boolean
boolean
string
string
Note
It's good practice to surround operators with a space. Exceptions are to not use spaces before or after the grouping operator ( ) and before the logical not ! operator.
Exercise 3.01: Implementing Expressions and Operators
In this exercise, you will interactively enter number, string, and comparison expressions into a web browser console window and review the results. Let's get started. Open the data-expressions.html document in your web browser:
- Open the web developer console window using your web browser.
In the next couple of steps, we will implement a few expressions using number data and arithmetic operators. Type the items on the lines beginning with the > symbol. The console window will show a response on the lines beginning with the < symbol.
- Write the following code to add two literal whole numbers:
> 200 + 200
\\output
< 400
- Write the following code to divide the literal whole numbers:
> 1000 / 4
\\Output
< 250
- Now, write the following code to divide a real number by a whole number:
> 150.75 / 3
\\Output
< 50.25
- Multiplication has a higher order of operator precedence, which is demonstrated with the following code:
> 100 + 100 * 2
\\Output
< 300
- We can use parentheses to change the order of operational precedence, as shown here:
> (100 + 100) * 2
\\Output
< 400
- To show the data type of a real number, we can use typeof, as shown here:
>typeof 987.123
\\Output
< "number"
- Let's try the following command:
> 123 456 789
\\Output
< Uncaught SyntaxError: Unexpected number
The output is a syntax error because you cannot have a number in this format with spaces (123 456 789 is not recognized as a number, but 123456789 is).
- We can compare two whole numbers using the > operator, as shown here:
> 100 > 200
\\Output
< false
- Similarly, we can compare two whole numbers using the < operator:
> 100 < 200
\\Output
< true
- Now, we can switch over and work with string data. Let's have a look at the output when we enter a literal string using double quotes as a delimiter:
> "Albert Einstein"
\\Output
< "Albert Einstein"
The next couple of snippets will show different examples of using literal strings.
- Using a literal string without delimiters would result in an error since JavaScript would not recognize such an input:
> Albert Einstein
\\Output
< Uncaught SyntaxError: Unexpected identifier
- You can use a literal string using double-quotes. Suppose you want to return the statement in double-quotes. You can place the double quotes in between a single quote:
> 'The quote "The only source of knowledge is experience" is attributed to Albert Einstein'
\\Output
< The quote "The only source of knowledge is experience" is attributed to Albert Einstein
- Use the \ escape character to use a delimiter. This turns special characters into string characters:
> "The quote \"The only source of knowledge is experience\" is attributed to Albert Einstein"
\\Output. Notice the escape character is removed.
< The quote "The only source of knowledge is experience" is attributed to Albert Einstein
- A non-mathematic number such as a phone number without delimiters will be converted into a number:
> 123-456-7890
\\Output. Expression converted to number
< -8223
- A non-mathematic number such as a phone number will appear as follows because we are using "":
> "123-456-7890"
\\Output
< "123-456-7890"
- We can also combine numbers and a literal string, as shown here:
> 100 - 10 + " Main Street"
\\Output.
< "90 Main Street"
When string is in expression JavaScript attempts to convert all other elements to a string.
- We can use the == operator to compare two strings with the same case:
> "Albert Einstein" == "Albert Einstein"
\\Output
< true
- Now, let's try comparing two strings with a different case:
> "Albert Einstein" == "ALBERT EINSTEIN"
\\Output
< false
- When we compare a number with a string with the same numeric value using the == operator, data type conversion takes place. This is shown in the following snippet:
> 100 == "100"
\\Output. Data type conversion takes place
< true
- If we want data type conversion to not take place before the comparison is made, we need to use the === operator, as shown here:
> 100 === "100"
\\Output. No data type conversion
< false
We used several operators and expressions in the preceding exercise. Real-world use cases of these operators and expressions would vary, depending on the type of applications being developed. However, the preceding exercise is a good starting point with regard to using these in actual programs. Note that, so far, the examples we used were using literal values. However, this may not always be the case in a real-world application. Often, values change dynamically while a program executes, and the use of variables in expressions becomes inevitable in such cases. The next section teaches you how you can use variables and constants in expressions.
Using Variables and Constants in Expressions
Variables and constants are symbolic names that are assigned to values. The value of a variable can be changed after it is assigned. The value that's assigned to a constant cannot be changed. Variables and constants involve the following items:
- Declaration keyword
- Name
- Assignment operator
- Expressions
- Data type
Variables and constants need to be declared before we can use them. For variables, there are two declaration keywords: var and let. For constants, the declaration keyword is const.
Variables and constants require a name. The JavaScript naming rules and conventions will be covered further in the chapter. The assignment operator is the single equals sign, =. The variable's data type is dynamic and is the same as the expression.
Variables do not need to be assigned a value when declared. A constant must be assigned a value when declared.
Have a look at the following examples of declaring a variable without assigning a value:
var firstName
var totalLikes
var errorMessage
var isSold
Variables that are not assigned a value still have a data type. That data type is named undefined. The typeof operator detects undefined data types.
Here are some examples of declaring a variable and assigning a value:
var firstName = "Albert"
var totalLikes = 50
var errorMessage = "Something terrible happened"
var isSold = false
Some examples of assigning a value to a variable that's been previously declared are as follows:
firstName = "Marie"
totalLikes = 50
errorMessage = "Something terrible happened"
isSold = false
Exercise 3.02: Working with Variables Using the Web Browser Console
In this exercise, you will use the web browser console window to work with variables. You will practice declaring variables, assigning values, and checking their data types. Let's get started:
- Use the variables.html file from https://packt.live/370myse in your web browser.
- Open the web developer console window using your web browser.
- Type the items onto the lines beginning with the > symbol. The console window will show a response on the lines beginning with the < symbol.
- Declare a variable named firstName:
> var firstName
\\Value is expressed as undefined
< undefined
- Write the data type of the variable:
>typeoffirstName
\\Output
< "undefined"
This is expected as we have not defined our variable with any value.
- Assign the string value Albert to the firstName variable:
>firstName = "Albert"
\\Output
< "Albert"
- To find out what data type our input is, use the typeof keyword, as shown here:
>typeoffirstName
\\Output
< "string"
As expected, our input is correctly identified as beginning a string.
- To find out what value our firstName variable is holding, we simply need to write the following code:
>firstName
\\Output
< "Albert"
Until now, we have used strings. In the next step, we will define a new variable and store a number value in it.
- Declare a variable and assign it to a number expression:
> var totalLikes = 50
\\Output. Console may express value when declared but before assigned
< undefined
- Write the value of totalLikes:
>totalLikes
\\Output
< 50
- To ascertain the data type, we will once again use typeof, as shown here:
>typeoftotalLikes
< "number"
So far, we haven't changed the values our variables are holding. We shall do this in the next step.
- Here is the code for changing the value held by totalLikes:
>totalLikes = totalLikes + 1
\\Output. New value is expressed
< 51
We can use the comparison operator, >, to compare the value our variable is holding with a reference value. This is done in the next step.
- Compare the value of totalLikes using the following code:
>totalLikes> 100
< false
The result is obviously false as the current value of totalLikes is 51.
Now, let's define a new variable and use Boolean expressions.
- Declare a variable and assign it to a Boolean expression:
> var isSold = false
\\Output. Console may express undefined data type when declared but before assigned.
< undefined
- Write the data type, like so:
>typeofisSold
< "boolean"
You have now interactively worked with declaring variables, assigned them values, and used them in expressions. We defined variables with different inputs such as strings, numbers, and Boolean values. You also used the typeof operator to reveal the data type of a variable. Now, we will progress to another important topic — functions.
Functions That Return Values
Functions may be written to return a value. In that case, we can use them in expressions. When we use a function, it is also called invoking the function.
To use a function in an expression, you need to include the function name, followed by parentheses. If the function requires input, it is placed inside the parentheses as valid expressions. These are called arguments. If more than one argument is needed, they are separated with commas.
These examples assume that the function will return a value.
Have a look at this example on expressing functions that do not require an argument:
getTotal()
isLoggedIn()
This example shows us expressing a function that has one argument expressed as a number literal:
getCelsiusFromFahrenheit(32)
This example shows us expressing a function that has multiple arguments using literal values:
getSearchResults("Pet Names", 25)
Finally, this example shows us expressing a function that has multiple arguments using variables:
var amount = 100000
var decimals = 2
var decimalSeparator = "."
var thousandsSeparator = ","
formatCurrency(amount, decimals, decimalSeparator, thousandsSeparator)
When you see a function in an expression, think of it as representing a value.
Exercise 3.03: Using Functions in Expressions
In this exercise, we will use a predefined function and then use it in expressions. This exercise will show how you can invoke, check, and return the data type, and use functions in expressions. For the purpose of this exercise, we will use a function defined as getDiceRoll. Let's get started:
- Open the use-functions.html document in your web browser.
- Open the web developer, console window, using your web browser.
The web page has a function named getDiceRoll. It returns the value of one rolled dice. It has one argument. The argument allows you to supply the number of dice to roll. Type the items on the lines beginning with the > symbol. The console window will show a response on the lines beginning with the <· symbol.
- Express the data type. Note that a function name without parentheses is used:
>typeofgetDiceRoll
\\Expressed as a function type. It also assures us that there is a function.
<·function
- Express the return value data type. Note that a function name with parentheses is used:
>typeofgetDiceRoll()
\\Function return value is a number. We do not see the actual value.
<·"number"
- Invoke the function using the following code:
>getDiceRoll()
\\Your value will be 1 to 6. Repeat a few times.
<·3
We can also invoke functions in math expressions.
- Invoke the function in a math expression:
> 100 * getDiceRoll()
\\Your value will be 100 to 600 Repeat a few times.
<·300
We can also invoke functions in a comparison expression.
- Invoke the function in a comparison expression:
>getDiceRoll() == 4
\\You may need to repeat a few times to get a true result.
<·true
So far, we haven't passed any arguments for our functions. However, remember that we do have the option to do so as our function is defined to accept a single argument. This argument defines the number of dices that will be rolled. Let's try passing an argument in the next step.
- Invoke and supply the argument for the number of dice to roll as 2:
>getDiceRoll(2)
\\You will receive values from 2 to 12.
<·11
Functions are critical to JavaScript programming. To get you started, we have only shown how you can use predefined functions. You will learn to write your own functions later in this chapter. However, you may come across scenarios in which you may have to use functions that have already been created. This exercise was a good starting point in showing you how this can be done.
The Object Data Type
JavaScript is designed around object data, thus making it important to understand. There are JavaScript objects that have been ready-made for us to use and you, as a programmer, will create objects. In either case, JavaScript objects are composed of properties and methods:
Property: A value that has an assigned named. Together, they are often called a name/value pair. Values can be any type, that is, data, a number, a string, a Boolean, or an object. Property values can be changed dynamically.
Method: A function that performs an action.
Ready-Made Objects
JavaScript has ready-made objects that we can use to help us begin to learn how to program. There are many useful objects built into JavaScript. Web browsers provide a collection of objects called the Document Object Model (DOM).
Some examples of ready-made objects are as follows:
- window is an object in DOM. It has access to the web browser's open window. Often considered a top-level DOM object containing other web browser-created objects as its properties, it has methods for setting timer events and printing.
- console is an object in DOM. It provides the ability to output to the web browser console window. It is also a property of the window object.
- document is an object in DOM. It has access to a web page's HTML elements, styles, and content. It is also a property of the window object.
- location is an object in DOM. It has information about the current URL. It is a property of the window object.
- Math is a built-in object. It consists of math constants such as Pi, and functions such as rounding.
- Date is a built-in object. It provides calendar date and time operations.
Exercise 3.04: Working with Ready-Made Objects
In this exercise, we will experiment with the properties and methods of ready-made objects that are available to JavaScript in the web browser. We will use the random, round, ceil, and floor methods to invoke a math object from a pre-defined object. Let's get started:
- Open the objects-ready-made.html document in your web browser.
- Open the web developer console window using your web browser.
- First, we will start with the web browser document object. Type the items on the lines beginning with the > symbol. The console window will show a response on the lines beginning with the <· symbol.
- Display the document object title property:
>document.title
\\Output
<< "JavaScript Data and Expression Practice | Packt Publishing"
- Now, display the document object doctype property:
>document.doctype
\\Output
<<!doctype html>
- Display the document object lastModified property:
>document.lastModified
\\Your output may have a different time and date value.
< "09/09/2019 21:58:25"
- Declare a variable and assign it to the HTMLElement object variable using the document object getElementById method:
> var pageHeadEle = document.getElementById('page-heading')
\\Console may express undefined data type when declared but before assigned.
<·undefined
- Display the pageHeadEleHTMLElement object:
>pageHeadEle
\\Output
<<div id="page-heading" class="heading-section">
<h1 class="center-text">JavaScript Data and Expression Practice</h1>
</div>
- Write the pageHeadEle object innerHTML property:
>pageHeadEle.innerHTML
\\Output
<·"
<h1 class="center-text">JavaScript Data and Expression Practice</h1>
"
- Now, let's look at the JavaScript built-in Math object. Write the Math object PI property:
>Math.PI
\\Output
< 3.141592653589793
- Invoke the random method for the Math object:
>Math.random()
< 0.9857480203668554
The Math.random() method returns a random number from the range 0 and 1, both inclusive. It returns a different value with every call.
- Invoke the random method for the Math object:
>Math.random()
<·0.3588305599787365
- Invoke the random method for the Math object:
>Math.random()
<·0.45663802022566413
- Use the Math object's round method:
>Math.round(10.5)
<·11
- Use the Math object's round method:
>Math.round(10.4)
<·10
The Math.round() method returns the number after rounding it off to its nearest integer.
- Use the Math object's ceil method:
>Math.ceil(10.5)
<·11
The Match.ceil() method returns the next smallest integer value that is greater than, or equal to, the given argument.
- Use the Math object's ceil method:
>Math.ceil(10.4)
<·11
- Use the Math object's floor method:
>Math.floor(10.4)
<·10
The Math.floor() method returns the previous largest integer value that is less than, or equal to, the given argument.
- Use the Math object's floor method:
>Math.floor(10.6)
<·10
- This is the expression we use to get a random dice value. The floor method argument is an expression, that is, Math.random() * 6. Its result is added to 1:
>Math.floor(Math.random() * 6) + 1
\\Output
< 1
There are many ready-made objects available in JavaScript. They are used just like other functions and variables, except we call the functions as methods and the variables as properties.
Self-Made Objects
You often have to create objects when developing real-world applications. They help you organize a set of data and functions that work together. Think about what properties and methods you may use for a stopwatch object.
You can see that we named the properties and methods as follows:
- elapsedTime is a property with a data type number. It displays the seconds that have elapsed since timing started.
- resultsHistory is a property data type object. It displays a list of previous timings.
- isTiming is a property data type Boolean. It displays the state of its timing.
- isPaused is a property data type Boolean. It displays the state if paused.
- start is a method data type function. It starts timing and sets elapsedTime to 0.
- pause is a method data type function. It pauses the timing.
- resume is a method data type function. It resumes the timing.
- stop is a method data type function. It stops timing and adds the result to resultsHistory.
Object Dot Notation
To reference object properties and methods, you use dot notation. This is the object name, followed by a period, and then the name of the property or method. Let's use the stopWatch object as an example:
stopWatch.elapsedTime
stopWatch.start()
stopWatch.start()
stopWatch.stop()
Methods require parentheses after the name. If the method requires data input, the data is placed inside the parentheses.
The Array Object
Arrays are objects that represent a list of values. Each item in the list is called an element. To initialize an array, you can set it to an array literal. An array literal is a comma-separated list of expressions enclosed in square brackets, like so:
["Saab", "Ford", "BMW", "GM"]
Elements in arrays can be different data types. Often, all the elements are the same data type:
["Milk", false, 123, document, "Gold", -.9876]
Elements in a literal array can be expressions, but they are evaluated and only the expression values are stored:
[price - cost, Math.random(), document.title, someVariable / 2]
Variables and object properties can contain arrays:
let todoList = [
"Wash Laundry",
"Clean Silver",
"Write Letters",
"Purchase Groceries",
"Retrieve Mail",
"Prepare Dinner"
]
game.scores = [120, 175, 145, 200]
Array elements can be arrays:
notes = [
[
"Wash Laundry",
"Clean Silver",
"Write Letters"
], 123, "999-999-9999"
]
The array objects with useful properties and methods are as follows:
- length is a property with a number data-type that displays the number of items in the array.
- push is a method with a number data-type that appends an element and returns the new length.
- unshift is a method with a number data-type that prepends an element and returns the new length.
- shift is a method with a mixed data-type that removes the first element and returns the removed element's value.
- pop is a method with a mixed data-type that removes the last element to return the removed element's value.
- concat is a method with a function data-type that merges two or more arrays to return a new array.
Using the Console Object
The console object has a method called log that we can use to test expressions in a JavaScript program. It takes an unlimited number of expressions separated by commas. All the expressions we enter into the console window would work with the console.log method. It evaluates the expressions and returns their results in the console. Multiple expressions are separated by a space.
The console.log method will be used in the upcoming exercises. Let's have a look at its syntax:
console.log(expression 1[, expression 2][, expression n])
Here are some examples of the console.log method:
console.log("Odd number count started!");
console.log("Iteration:", i);
console.log("Number:", number);
console.log(oddsCount + " odd numbers found!");
console.log(document);