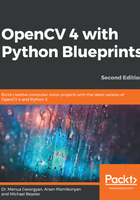
Detecting features in an image with SURF
In the remainder of this chapter, we will make use of the SURF detector. The SURF algorithm can be roughly divided into two distinct steps, which are detecting points of interest and formulating a descriptor.
SURF relies on the Hessian corner detector for interest point detection, which requires the setting of a minimal minhessianThreshold. This threshold determines how large the output from the Hessian filter must be in order for a point to be used as an interesting point.
When the value is larger, fewer interest points are obtained, but they are theoretically more salient and vice versa. Feel free to experiment with different values.
In this chapter, we will choose a value of 400, as we did earlier in FeatureMatching.__init__, where we created a SURF descriptor with the following code snippet:
self.f_extractor = cv2.xfeatures2d_SURF.create(hessianThreshold=400)
The keypoints in the image can be obtained in a single step, which is given as follows:
key_query = self.f_extractor.detect(img_query)
Here, key_query is a list of instances of cv2.KeyPoint and has the length of the number of detected keypoints. Each KeyPoint contains information about the location (KeyPoint.pt), the size ( KeyPoint.size ), and other useful information about our point of interest.
We can now easily draw the keypoints using the following function:
img_keypoints = cv2.drawKeypoints(img_query, key_query, None,
(255, 0, 0), 4)
cv2.imshow("keypoints",img_keypoints)
Depending on an image, the number of detected keypoints can be very large and unclear when visualized; we check it with len(keyQuery). If you care only about drawing the keypoints, try setting min_hessian to a large value until the number of returned keypoints provides a good illustration.
Note that SURF is protected by patent laws. Therefore, if you wish to use SURF in a commercial application, you will be required to obtain a license.
In order to complete our feature extraction algorithm, we need to obtain descriptors for our detected keypoints, which we will do in the next section.