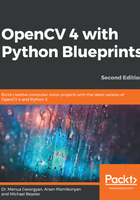
上QQ阅读APP看书,第一时间看更新
Running the app – the main() function routine
To run our app, we will need to execute the main() function routine. The following steps show us the execution of main() routine:
- The function first accesses the webcam with the VideoCapture method by passing 0 as an argument, which is a reference to the default webcam. If it can not access the webcam, the app will be terminated:
import cv2 as cv
from feature_matching import FeatureMatching
def main():
capture = cv.VideoCapture(0)
assert capture.isOpened(), "Cannot connect to camera"
- Then, the desired frame size and frame per second of the video stream is set. The following snippet shows the code for setting the frame size and frame per second of the video:
capture.set(cv.CAP_PROP_FPS, 10)
capture.set(cv.CAP_PROP_FRAME_WIDTH, 640)
capture.set(cv.CAP_PROP_FRAME_HEIGHT, 480)
- Next, an instance of the FeatureMatching class is initialized with a path to a template (or training) file that depicts the object of interest. The following code shows the FeatureMatching class:
matching = FeatureMatching(train_image='train.png')
- After that, to process the frames from the camera, we create an iterator from the capture.read function, which will terminate when the function fails to return frame ((False,None)). This can be seen in the following code block:
for success, frame in iter(capture.read, (False, None)):
cv.imshow("frame", frame)
match_succsess, img_warped, img_flann = matching.match(frame)
In the previous code block, the FeatureMatching.match method processes the BGR image (capture.read returns frame in BGR format). If the object is detected in the current frame, the match method will report match_success=True and return the warped image as well as the image that illustrates the matches—img_flann.
Let's move on and display the results in which our match method will return.