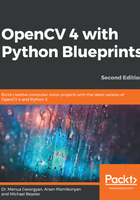
Distinguishing between different causes of convexity defects
The trick is to look at the angle between the farthest point from the convex hull point within the defect (farthest_pt_index) and the start and endpoints of the defect (start_index and end_index, respectively), as illustrated in the following screenshot:
In the previous screenshot, the orange markers serve as a visual aid to center the hand in the middle of the screen, and the convex hull is outlined in green. Each red dot corresponds to the point farthest from the convex hull (farthest_pt_index) for every convexity defect detected. If we compare a typical angle that belongs to two extended fingers (such as θj) to an angle that is caused by general hand geometry (such as θi), we notice that the former is much smaller than the latter.
This is obviously because humans can spread their fingers only a little, thus creating a narrow angle made by the farthest defect point and the neighboring fingertips. Therefore, we can iterate over all convexity defects and compute the angle between the said points. For this, we will need a utility function that calculates the angle (in radians) between two arbitrary—a list like vectors, v1 and v2, as follows:
def angle_rad(v1, v2): return np.arctan2(np.linalg.norm(np.cross(v1, v2)),
np.dot(v1, v2))
This method uses the cross product to compute the angle, rather than doing it in the standard way. The standard way of calculating the angle between two vectors v1 and v2 is by calculating their dot product and dividing it by the norm of v1 and the norm of v2. However, this method has two imperfections:
- You have to manually avoid division by zero if either the norm of v1 or the norm of v2 is zero.
- The method returns relatively inaccurate results for small angles.
Similarly, we provide a simple function to convert an angle from degrees to radians, illustrated here:
def deg2rad(angle_deg): return angle_deg/180.0*np.pi
In the next section, we'll see how to classify hand gestures based on the number of extended fingers.