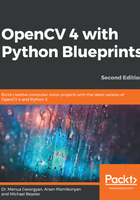
上QQ阅读APP看书,第一时间看更新
Running the app
To run the application, we will turn to the chapter1.py script. Follow these steps to do so:
- We first start by importing all the necessary modules:
import wx
import cv2
import numpy as np
- We will also have to import a generic GUI layout (from wx_gui) and all the designed image effects (from tools):
from wx_gui import BaseLayout
from tools import apply_hue_filter
from tools import apply_rgb_filters
from tools import load_img_resized
from tools import spline_to_lookup_table
from tools import cartoonize
from tools import pencil_sketch_on_canvas
- OpenCV provides a straightforward way to access a computer's webcam or camera device. The following code snippet opens the default camera ID (0) of a computer using cv2.VideoCapture:
def main(): capture = cv2.VideoCapture(0)
- In order to give our application a fair chance to run in real time, we will limit the size of the video stream to 640 x 480 pixels:
capture.set(cv2.CAP_PROP_FRAME_WIDTH, 640)
capture.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
- Then, the capture stream can be passed to our GUI application, which is an instance of the FilterLayout class:
# start graphical user interface
app = wx.App()
layout = FilterLayout(capture, title='Fun with Filters')
layout.Center()
layout.Show()
app.MainLoop()
After we create FilterLayout, we center the layout, so it appears in the center of the screen. And we call Show() to actually show the layout. Finally, we call app.MainLoop(), so the application starts working, receiving, and processing events.
The only thing left to do now is to design the said GUI.