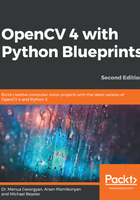
Using an optimized version of a Gaussian blur
A Gaussian blur is basically a convolution with a Gaussian function. Well, one of the features of convolutions is their associative property. This means that it does not matter whether we first invert the image and then blur it, or first blur the image and then invert it.
If we start with a blurred image and pass its inverse to the dodge function, then within that function the image will be inverted again (the 255-mask part), essentially yielding the original image. If we get rid of these redundant operations, the optimized convert_to_pencil_sketch function will look like this:
def convert_to_pencil_sketch(rgb_image):
gray_image = cv2.cvtColor(rgb_image, cv2.COLOR_RGB2GRAY)
blurred_image = cv2.GaussianBlur(gray_image, (21, 21), 0, 0)
gray_sketch = cv2.divide(gray_image, blurred_image, scale=256)
return cv2.cvtColor(gray_sketch, cv2.COLOR_GRAY2RGB)
For kicks and giggles, we want to lightly blend our transformed image (img_sketch) with a background image (canvas) that makes it look as though we drew the image on a canvas. So, before returning, we would like to blend with canvas if it exists:
if canvas is not None:
gray_sketch = cv2.multiply(gray_sketch, canvas, scale=1 / 256)
We name our final function pencil_sketch_on_canvas, and it looks like this (together with optimizations):
def pencil_sketch_on_canvas(rgb_image, canvas=None):
gray_image = cv2.cvtColor(rgb_image, cv2.COLOR_RGB2GRAY)
blurred_image = cv2.GaussianBlur(gray_image, (21, 21), 0, 0)
gray_sketch = cv2.divide(gray_image, blurred_image, scale=256)
if canvas is not None:
gray_sketch = cv2.multiply(gray_sketch, canvas, scale=1 / 256)
return cv2.cvtColor(gray_sketch, cv2.COLOR_GRAY2RGB)
This is just our convert_to_pencil_sketch function, with the optional canvas argument that can add an artistic touch to the pencil sketch.
And we're done! The final output looks like this:
Let's take a look at how to generate a warming and cooling filter in the next section, where you'll learn how to use lookup tables for image manipulation.