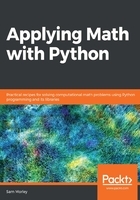
Preface
Python is a powerful and flexible programming language that is fun and easy to learn. It is the programming language of choice for many professionals, hobbyists, and scientists. The power of Python comes from its large ecosystem of packages and friendly community, and from its ability to communicate seamlessly with compiled extension modules. This means that Python is ideal for solving problems of all kinds, and mathematical problems in particular.
Mathematics is usually associated with calculations and equations, but in reality, these are very small parts of a much larger subject. At its core, mathematics is about solving problems, and the logical, structured approach to solutions. Once you explore past the equations, calculations, derivatives, and integrals, you discover a vast world of beautiful, elegant structures.
This book is an introduction to solving mathematical problems using Python. It provides an introduction to some of the basic concepts from mathematics – and how to use Python to work with these concepts – and templates for solving a variety of mathematical problems across a large number of topics within mathematics. The first few chapters focus on core skills such as working with NumPy arrays, plotting, calculus, and probability. These topics are very important throughout mathematics, and act as the foundation for the rest of the book. In the remaining chapters, we discuss more practical problems, covering topics such as data analysis and statistics, networks, regression and forecasting, optimization, and game theory. We hope that this book provides a basis for solving mathematical problems and the tools for you to further explore the world of mathematics.
Who this book is for
Readers will need to have a basic knowledge of Python. We don't assume any knowledge of mathematics, although readers who are familiar with some basic mathematical concepts will better understand the context and details of the techniques we discuss.
What this book covers
Chapter 1, Basic Packages, Functions, and Concepts, introduces some of the basic tools and concepts that will be needed in the rest of the book, including the main Python packages for mathematical programming, NumPy and SciPy.
Chapter 2, Mathematical Plotting with Matplotlib, covers the basics of plotting with Matplotlib, which is useful when solving almost all mathematical problems.
Chapter 3, Calculus and Differential Equations, introduces topics from calculus such as differentiation and integration, and some more advanced topics such as ordinary and partial differential equations.
Chapter 4, Working with Randomness and Probability, introduces the fundamentals of randomness and probability, and how to use Python to explore these ideas.
Chapter 5, Working with Trees and Networks, covers working with trees and networks (graphs) in Python using the NetworkX package.
Chapter 6, Working with Data and Statistics, gives various techniques for handling, manipulating, and analyzing data using Python.
Chapter 7, Regression and Forecasting, describes various techniques for modeling data and predicting future values using the Statsmodels package and scikit-learn.
Chapter 8, Geometric Problems, demonstrates various techniques for working with geometric objects in Python using the Shapely package.
Chapter 9, Finding Optimal Solutions, introduces optimization and game theory, which use mathematical methods to find the best solutions to problems.
Chapter 10, Miscellaneous Topics, covers an assortment of situations that you might encounter while solving mathematical problems using Python.
To get the most out of this book
The only requirement throughout this book is a recent version of Python, at least Python 3.6, but a higher version is preferable. Some readers might prefer to use the Anaconda distribution of Python, which comes with many of the packages and tools required in this book. If this is the case, you should use the conda package manager to install the packages. Python is supported on all major operating systems – Windows, macOS, and Linux – and on many platforms. The following table covers the main libraries and their versions used at the time of writing this book:

If you are using the digital version of this book, we advise you to type the code yourself or access the code via the GitHub repository (link available in the next section). Doing so will help you avoid any potential errors related to the copying and pasting of code.
Some readers may prefer to work through the code samples in this book in a Jupyter notebook rather than in a simple Python file. There are one or two places in this book where you may need to repeat plotting commands. These places are marked in the instructions.
Download the example code files
You can download the example code files for this book from your account at www.packt.com. If you purchased this book elsewhere, you can visit www.packtpub.com/support and register to have the files emailed directly to you.
You can download the code files by following these steps:
- Log in or register at www.packt.com.
- Select the Support tab.
- Click on Code Downloads.
- Enter the name of the book in the Search box and follow the onscreen instructions.
Once the file is downloaded, please make sure that you unzip or extract the folder using the latest version of:
- WinRAR/7-Zip for Windows
- Zipeg/iZip/UnRarX for Mac
- 7-Zip/PeaZip for Linux
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/Applying-Math-with-Python. In case there's an update to the code, it will be updated on the existing GitHub repository.
We also have other code bundles from our rich catalog of books and videos available athttps://github.com/PacktPublishing/. Check them out!
Code in Action
Code in Action videos for this book can be viewed at https://bit.ly/2ZQcwIM.
Conventions used
There are a number of text conventions used throughout this book.
CodeInText: Indicates code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles. Here is an example: "Thedecimal package also provides aContext object, which allows fine-grained control over the precision, display, and attributes ofDecimal objects."
A block of code is set as follows:
from decimal import getcontext
ctx = getcontext()
num = Decimal('1.1')
num**4 # Decimal('1.4641')
ctx.prec = 4 # set new precision
num**4 # Decimal('1.464')
When we wish to draw your attention to a particular part of a code block, the relevant lines or items are set in bold:
from numpy import linalg
A = np.array([[3, -2, 1], [1, 1, -2], [-3, -2, 1]])
b = np.array([7, -4, 1])
Any command-line input or output is written as follows::
python3.8 -m pip install numpy scipy
Bold: Indicates a new term, an important word, or words that you see on screen. For example, words in menus or dialog boxes appear in the text like this. Here is an example: "Select System info from the Administration panel."
Sections
In this book, you will find several headings that appear frequently (Getting ready, How to do it..., How it works..., There's more..., and See also).
To give clear instructions on how to complete a recipe, use these sections as follows:
Getting ready
This section tells you what to expect in the recipe and describes how to set up any software or any preliminary settings required for the recipe.
How to do it…
This section contains the steps required to follow the recipe.
How it works…
This section usually consists of a detailed explanation of what happened in the previous section.
There's more…
This section consists of additional information about the recipe in order to make you more knowledgeable about the recipe.
See also
This section provides helpful links to other useful information for the recipe.
Get in touch
Feedback from our readers is always welcome.
General feedback: If you have questions about any aspect of this book, mention the book title in the subject of your message and email us at customercare@packtpub.com.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you would report this to us. Please visit www.packtpub.com/support/errata, selecting your book, clicking on the Errata Submission Form link, and entering the details.
Piracy: If you come across any illegal copies of our works in any form on the Internet, we would be grateful if you would provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in, and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Reviews
Please leave a review. Once you have read and used this book, why not leave a review on the site that you purchased it from? Potential readers can then see and use your unbiased opinion to make purchase decisions, we at Packt can understand what you think about our products, and our authors can see your feedback on their book. Thank you!
For more information about Packt, please visit packt.com.