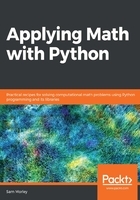
Basic mathematical functions
Basic mathematical functions appear in many applications. For example, logarithms can be used to scale data that grows exponentially to give linear data. The exponential function and trigonometric functions are common fixtures when working with geometric information, the gamma function appears in combinatorics, and the Gaussian error function is important in statistics.
The math module in the Python Standard Library provides all of the standard mathematical functions, along with common constants and some utility functions, and it can be imported using the following command:
import math
Once it's imported, we can use any of the mathematical functions that are contained in this module. For instance, to find the square root of a non-negative number, we would use the sqrt function from math:
import math
math.sqrt(4) # 2.0
Attempting to use the sqrt function with a negative argument will raise a ValueError. The square root of a negative number is not defined for this sqrt function, which deals only with real numbers. The square root of a negative number—this will be a complex number—can be found using the alternative sqrt function from the cmath module in the Python Standard Library.
The trigonometric functions, sine, cosine, and tangent, are available under their common abbreviations sin, cos, and tan, respectively, in the math module. Thepi constant holds the value of π, which is approximately 3.1416:
theta = pi/4
math.cos(theta) # 0.7071067811865476
math.sin(theta) # 0.7071067811865475
math.tan(theta) # 0.9999999999999999
The inverse trigonometric functions are named acos, asin, and atan in the math module:
math.asin(-1) # -1.5707963267948966
math.acos(-1) # 3.141592653589793
math.atan(1) # 0.7853981633974483
The log function in the math module performs logarithms. It has an optional argument to specify the base of the logarithm (note that the second argument is positional only). By default, without the optional argument, it is the natural logarithm with base e. The econstant can be accessed using math.e:
math.log(10) # 2.302585092994046
math.log(10, 10) # 1.0
The math module also contains the function gamma, which is the gamma function, and the function erf, the Gaussian error function, which is important in statistics. Both of these functions are defined by integrals. The gamma function is defined by the integral

and the error function is defined by

The integral in the definition of the error function cannot be evaluated using calculus, and instead must be computed numerically:
math.gamma(5) # 24.0
math.erf(2) # 0.9953222650189527
In addition to standard functions such as trigonometric functions, logarithms, and exponential functions, the math module contains various number of theoretic and combinatorial functions. These include the functions comb and factorial, which are useful in a variety of applications. The comb function called with arguments n and k returns the number of ways to choose k items from a collection of n without repeats if order is not important. For example, picking 1 then 2 is the same as picking 2 then 1. This number is sometimes written nCk. The factorial called with argument n returns the factorial n! = n(n-1)(n-2)…1:
math.comb(5, 2) # 10
math.factorial(5) # 120
Applying the factorial to a negative number raises a ValueError. The factorial of an integer n, coincides with the value of the gamma function at n + 1; that is,

The math module also contains a function that returns the greatest common divisor of its arguments called gcd. The greatest common divisor of a and b is the largest integer k such that k divides both a and b exactly:
math.gcd(2, 4) # 2
math.gcd(2, 3) # 1
There are also a number of functions for working with floating-point numbers. The fsum function performs addition on an iterable of numbers and keeps track of the sums each step to reduce the error in the result. This is nicely illustrated by the following example:
nums = [0.1]*10 # list containing 0.1 ten times
sum(nums) # 0.9999999999999999
math.fsum(nums) # 1.0
The isclosefunctionreturnsTrue if the difference between the arguments is smaller than the tolerance. This is especially useful in unit tests, where there may be small variations in results based on machine architecture or data variability.
Finally, the floor and ceilfunctionsfrommath provide the floor and ceiling of their argument. Thefloor of a number xis the largest integerf withf ≤ x, and theceiling ofx is the smallest integerc withx ≤ c. These functions are useful when converting between a float obtained by dividing one number by another and an integer.
The math module contains functions that are implemented in C (assuming you are running CPython), and so are much faster than those implemented in Python. This module is a good choice if you need to apply a function to a relatively small collection of numbers. If you want to apply these functions to a large collection of data simultaneously, it is better to use their equivalents from the NumPy package, which are more efficient for working with arrays. In general, if you have imported the NumPy package already, then it is probably best to always use NumPy equivalents of these functions to limit the chance of error.