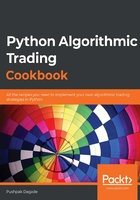
Creating a datetime object from a string
This recipe demonstrates the conversion of well-formatted strings into datetime objects. This finds application in reading timestamps from a file. Also, this is helpful while receiving timestamps as JSON data over web APIs.
How to do it…
Execute the following steps for this recipe:
- Import the necessary modules from the Python standard library:
>>> from datetime import datetime
- Create a string representation of timestamp with date, time, and time zone. Assign it to now_str:
>>> now_str = '13-1-2021 15:53:39 +05:30'
- Convert now_str to now, a datetime.datetime object. Print it:
>>> now = datetime.strptime(now_str, "%d-%m-%Y %H:%M:%S %z")
>>> print(now)
We get the following output:
2021-01-13 15:53:39+05:30
- Confirm that now is of the datetime type:
>>> print(type(now))
We get the following output:
<class 'datetime.datetime'>
How it works...
In step 1, you import the datetime class from the datetime module. In step 2, you create a string holding a valid timestamp and assign it to a new attribute, now_str. The datetime module has a strptime() method which can convert a string holding a valid timestamp in a specific format to a datetime object. In step 3, you convert now_str, a string in the format DD-MM-YYYY HH:MM:SS +Z, to now. In step 4, you confirm that now is indeed an object of the datetime type. The directives used in step 3 are the same as those described in the Converting a datetime object to a string recipe.
There's more
When reading a string into a datetime object, the entire string should be consumed with appropriate directives. Consuming a string partially will throw an exception, as shown in the following code snippet. The error message shows what data was not converted and can be used to fix the directives provided to the strptime() method.
Try to convert now_str to a datetime object using strptime() method. Pass a string with directives for only the date part of the string. Note the error:
>>> now = datetime.strptime(now_str, "%d-%m-%Y")
The output is as follows:
# Note: It's expected to have an error below
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-96-dc92a0358ed8> in <module>
----> 1 now = datetime.strptime(now_str, "%d-%m-%Y")
2 # Note: It's expected to get an error below
/usr/lib/python3.8/_strptime.py in _strptime_datetime(cls, data_string, format)
566 """Return a class cls instance based on the input string and the
567 format string."""
--> 568 tt, fraction, gmtoff_fraction = _strptime(data_string, format)
569 tzname, gmtoff = tt[-2:]
570 args = tt[:6] + (fraction,)
/usr/lib/python3.8/_strptime.py in _strptime(data_string, format)
350 (data_string, format))
351 if len(data_string) != found.end():
--> 352 raise ValueError("unconverted data remains: %s" %
353 data_string[found.end():])
354
ValueError: unconverted data remains: 15:53:39 +05:30