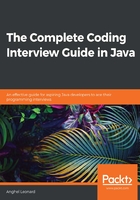
Preface
Java is a very popular language, featuring in a high number of IT job offers across a wide range of fields and industries. Since Java empowers billions of devices all over the world, it's become a very appealing technology to learn. However, learning Java is one thing; starting to develop a career in the Java field is something else. This book is dedicated to people who want to develop a Java career and want to ace Java-centric interviews.
With this book, you'll learn how to do the following:
- Solve the 220+ most popular Java coding interview problems in a contretemps fashion encountered in a wide range of companies, including top firms such as Google, Amazon, Microsoft, Adobe, and Flipkart.
- Collect the best techniques for solving a wide range of Java coding problems.
- Tackle brain-teasing algorithms meant to develop strong and fast logic abilities.
- Iterate the common non-technical interview questions that can make the difference between success and failure.
- Get an overall picture of what employers want from a Java developer.
By the end of this book, you will have a solid informational foundation for solving Java coding interview problems. The knowledge achieved from this book will give you high confidence in yourself to obtain your Java-centric dream job.
Who this book is for
The Complete Coding Interview Guide in Java is a comprehensive resource for those who are looking for a Java developer (or related) job and need to tackle coding problems in a contretemps fashion. It is especially dedicated to entry- and middle-level candidates.
What this book covers
Chapter 1, Where to Start and How to Prepare for the Interview, is a comprehensive guide that tackles the preparation process for a Java interview from zero to hire. More precisely, we want to highlight the main checkpoints that can ensure a smooth and successful career path ahead.
Chapter 2, What Interviews at Big Companies Look Like, talks about how interviews are conducted in the main Big Tech firms of Google, Amazon, Microsoft, Facebook, and Crossover.
Chapter 3, Common Non-Technical Questions and How To Answer Them, tackles the main aspects of the non-technical questions. This part of the interview is commonly carried out by a hiring manager or even an HR person.
Chapter 4, How to Handle Failures, discusses a delicate aspect of the interview – handling failures. The main purpose of this chapter is to show you how to identify the causes of failure and how to mitigate them in the future.
Chapter 5, How to Approach a Coding Challenge, covers the technical quizzes and coding challenge topics that are commonly referred to as the technical interview.
Chapter 6, Object-Oriented Programming, explains the most popular questions and problems concerning object-oriented programming encountered at Java interviews, including the SOLID principles and coding challenges such as Jukebox, Parking Lot, and Hash Table.
Chapter 7, Big O Analysis of Algorithms, provides the most popular metric for analyzing the efficiency and scalability of algorithms, the Big O notation, in the context of a technical interview.
Chapter 8, Recursion and Dynamic Programming, covers one of the favorite topics of interviewers – recursion and Dynamic Programming. Both of these topics work hand in hand with each other, so you have to be able to cover both.
Chapter 9, Bit Manipulation, explains the most important aspects of bit manipulation that you should know in a technical interview. Such problems are often encountered in interviews and they are not easy. In this chapter, you have 25 such coding challenges.
Chapter 10, Arrays and Strings, covers 29 popular problems involving strings and arrays.
Chapter 11, Linked Lists and Maps, teaches you the 17 most famous coding challenges that involve maps and linked lists encountered in interviews.
Chapter 12, Stacks and Queues, explains the 11 most popular interview coding challenges involving stacks and queues. Mainly, you have to learn how to provide a stack/queue implementation from scratch and how to tackle coding challenges via the Java built-in implementations.
Chapter 13, Trees and Graphs, covers one of the most tricky topics in interviews – trees and graphs. While there are tons of problems related to these two topics, only a handful of them are actually encountered in interviews. It is therefore very important to give a high priority to the most popular problems concerning trees and graphs.
Chapter 14, Sorting and Searching, covers the most popular sorting and searching algorithms encountered in technical interviews. We will cover sorting algorithms such as Merge Sort, Quick Sort, Radix Sort, Heap Sort, and Bucket Sort, and searching algorithms such as Binary Search. By the end of this chapter, you should be able to tackle a wide range of problems that involve sorting and searching algorithms.
Chapter 15, Mathematics and Puzzles, talks about a controversial topic in interviews: mathematics and puzzle problems. A significant number of companies consider that these kinds of problems should not be part of a technical interview, while other companies still regard this topic as relevant for interviews.
Chapter 16, Concurrency, covers the most popular questions about Java concurrency (multithreading) that occur in general interviews involving the Java language.
Chapter 17, Functional-Style Programming, examines the most popular questions about Java functional-style programming. We cover key concepts, lambdas, and streams.
Chapter 18, Unit Testing, talks about unit-testing interview problems that you may encounter if you apply for a position such as a developer or software engineer. Of course, if you are looking for a tester (manual/automation) position, then this chapter may represent just another perspective on testing. Therefore, do not expect to see questions here specific to manual/automation tester positions.
Chapter 19, System Scalability, provides the widest range of scalability interview questions you may be asked during a junior/middle-level interview for a position such as a web application software architect, Java architect, or software engineer.
To get the most out of this book
All you need is Java (preferably Java 8+) and your favorite IDE (NetBeans, IntelliJ IDEA, Eclipse, and so on).
I also strongly recommend that readers consult the Java Coding Problems book, also from Packt, to improve your skills further.
Download the example code files
You can download the example code files for this book from your account at www.packt.com. If you purchased this book elsewhere, you can visit www.packtpub.com/support and register to have the files emailed directly to you.
You can download the code files by following these steps:
- Log in or register at www.packt.com.
- Select the Support tab.
- Click on Code Downloads.
- Enter the name of the book in the Search box and follow the onscreen instructions.
Once the file is downloaded, please make sure that you unzip or extract the folder using the latest version of:
- WinRAR/7-Zip for Windows
- Zipeg/iZip/UnRarX for Mac
- 7-Zip/PeaZip for Linux
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/The-Complete-Coding-Interview-Guide-in-Java. In case there's an update to the code, it will be updated on the existing GitHub repository.
We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!
Download the color images
We also provide a PDF file that has color images of the screenshots/diagrams used in this book. You can download it here:
https://static.packt-cdn.com/downloads/9781839212062_ColorImages.pdf
Conventions used
There are a number of text conventions used throughout this book.
Code in text: Indicates code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles. Here is an example: 'The Triangle, Rectangle, and Circle classes implement the Shape interface and override the draw() method to draw the corresponding shape."
A block of code is set as follows:
public static void main(String[] args) {
Shape triangle = new Triangle();
Shape rectangle = new Rectangle();
Shape circle = new Circle();
triangle.draw();
rectangle.draw();
circle.draw();
}
When we wish to draw your attention to a particular part of a code block, the relevant lines or items are set in bold:
public static void main(String[] args) {
Shape triangle = new Triangle();
Shape rectangle = new Rectangle();
Shape circle = new Circle();
triangle.draw();
rectangle.draw();
circle.draw();
}
Bold: Indicates a new term, an important word, or words that you see on screen. For example, words in menus or dialog boxes appear in the text like this. Here is an example: "However, this approach does not work for the third case, 339809 (1010010111101100001)."
Tips or important notes
Appear like this.
Get in touch
Feedback from our readers is always welcome.
General feedback: If you have questions about any aspect of this book, mention the book title in the subject of your message and email us at customercare@packtpub.com.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you would report this to us. Please visit www.packtpub.com/support/errata, selecting your book, clicking on the Errata Submission Form link, and entering the details.
Piracy: If you come across any illegal copies of our works in any form on the internet, we would be grateful if you would provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in, and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Reviews
Please leave a review. Once you have read and used this book, why not leave a review on the site that you purchased it from? Potential readers can then see and use your unbiased opinion to make purchase decisions, we at Packt can understand what you think about our products, and our authors can see your feedback on their book. Thank you!
For more information about Packt, please visit packt.com.