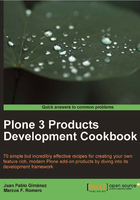
Creating a test suite with ArchGenXML
Up till now we have created and run different kinds of test suites based on paster-generated products. Nevertheless ArchGenXML can also create all the necessary boilerplate we need for testing.
We are going to use the same UML model and product that we developed in the Creating a model and Generating code tasks in the previous chapter. If you didn't read that one, you can use the example code available for download. We'll also use ArgoUML in the next examples.
Getting ready
In the previous chapter, we created two products (one with ArchGenXML in the Generating code recipe and the other one with paster in Installation of the product) and merged them in an egg directory structure. If we plan to model tests suites with a UML application, we need to remove the tests
module from the paster-created package.
In your instance folder, run the following command to remove tests.py
:
rm src/Products.poxContentTypes/Products/poxContentTypes/tests.py
In Windows, run:
del src\Products.poxContentTypes\Products\poxContentTypes\tests.py
How to do it...
Launch ArgoUML or your favourite UML program, and then open the model you have been working with (poxContentTypes.zargo
in our case, available also for download).
- Create a new package (third icon in the toolbar) and name it
tests
. - Add a new class to it (fourth icon in the toolbar) and name it
poxContentTypesTestCase
. Make sure the class is inside the package. - Apply the <<plone_testcase>> stereotype to
poxContentTypesTestCase
class. You can do this in the Stereotype tab in the bottom right pane. - Create a new class inside the
tests
package, name ittestXNewsItem
, and then apply the <<doc_testcase>> stereotype to it. We could have used <<testcase>> stereotype, but we prefer using doctests. Make this class derive frompoxContentTypesTestCase
with a generalization arrow (ninth icon in the toolbar). - Optionally, you can add a
testSetup
class with a <<setup_testcase>> stereotype. Make it also derive frompoxContentTypesTestCase
with a generalization arrow. This will make ArchGenXML create a newtestSetup
module with some general checks (the correct installation of a tool, a content type, skins, workflows, among others) that you can take as examples of how to perform your own tests.
After these steps, you should have arrived at a model like the one shown below:

Don't forget to save your work before running ArchGenXML to generate the code.
Go to your ArchGenXML models
folder (or where you placed the model) and run:
../bin/archgenxml ./poxContentTypes.zargo
If everything goes well, you'll see these lines as ArchGenXML output:

How it works...
You'll now have two new packages inside the poxContentTypes
product folder: doc
and tests
. The first one will have the doctest file textNewsItem.txt
and the second one will have all the test machinery, including a testSetup
module. Let's go through some of the files there.
The poxContentTypesTestCase
module contains the base test case class which we'll use for all the tests we are going to write:
... testcase = PloneTestCase.PloneTestCase ... PloneTestCase.setupPloneSite(products=PRODUCTS) class poxContentTypesTestCase(testcase): """Base TestCase for poxContentTypes.""" ... def test_suite(): from unittest import TestSuite, makeSuite suite = TestSuite() suite.addTest(makeSuite(poxContentTypesTestCase)) return suite ...
ArchGenXML test cases are based on (that is, inherited from) the PloneTestCase
class. This automatically turns them into integration tests, not unit tests. We can use them as a unit test, though. However, they will take longer to run.
testXNewsItem.py
file is the test file:
...
from Testing import ZopeTestCase
from Products.poxContentTypes.tests.poxContentTypesTestCase import poxContentTypesTestCase
# Import the tested classes
class testXNewsItem(poxContentTypesTestCase):
"""Test-cases for class(es) ."""
##code-section class-header_testXNewsItem #fill in your manual code here
##/code-section class-header_testXNewsItem
def afterSetUp(self):
pass
# Manually created methods
def test_suite():
from unittest import TestSuite
from Testing.ZopeTestCase.zopedoctest import ZopeDocFileSuite
from Testing.ZopeTestCase import ZopeDocFileSuite
##code-section test-suite-in-between #fill in your manual code here
##/code-section test-suite-in-between
s = ZopeDocFileSuite('testXNewsItem.txt', package='Products.poxContentTypes.doc', test_class=testXNewsItem)
...
This test case, created by ArchGenXML, inherits from the base poxContentTypesTestCase
class as defined in the previous module. In turn it will call a doctest (ZopedocFileSuite
) inside our product documentation folder: doc/testXNewsItem.txt
. If you open this file, you'll see a less useful test. However, you can replace it with the unit or integration tests, which we have already seen in Working with paster-generated test suites.
See also
- Creating a model
- Generating code
- Installation of the product
- Working with paster generated test suites