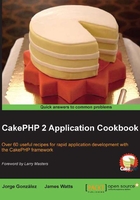
Parsing extensions
It's common for applications to use a file extension as part of the URL. This can sometimes help orientate the user to the type of content found in that location or for your application to easily deal with data types such as JSON or XML.
In this recipe, we'll look at how easy it is to parse extensions using the framework.
Getting ready
For this recipe, we'll use a books
controller from previous chapter, which will return a listing of books from the books
database table as a JSON response. So, find a file named BooksController.php
in app/Controller/
.
How to do it...
- First, add the following line to your
routes.php
file located inapp/Config/
:Router::parseExtensions('json');
- Then, we'll load the
RequestHandler
component in ourBooksController
class using the following code:public $components = array('RequestHandler');
- We'll also add a
listing()
method with the following code:public function listing() { $books = $this->Book->find('all', array('fields' => array('name', 'stock'))); $this->set(array( 'books' => Hash::extract($books, '{n}.Book'), '_serialize' => array('books') )); }
- Now, navigating to
/books/listing.json
in your browser will return a JSON listing of books similar to the following code:{'books':[{'title':'1984','author':'George Orwell'},{'title':'Neuromancer','author':'William Gibson'},{'title':'The Cuckoo\'s Egg','author':'Cliff Stoll'}]} { "books": [ { "name": "CakePHP Application", "stock": "2" }, { "name": "PHP for Beginners", "stock": "3" }, { "name": "Server Administration", "stock": "0" } ] }
How it works...
We first added a call to the static parseExtensions()
method of the Router
class. This method allows you to easily define extensions, which will be detected and handled automatically by the framework. By default, CakePHP provides both XML and JSON out of the box, but you can define any extension you like. You can also define multiple extensions by simply specifying the different extensions in the method call. An example can be seen in the following code:
Router::parseExtensions('xml', 'json');
Next, we included the RequestHandler
component in our BooksController
class, as this handles the internal logic to process the extension routing. You must include this component in any controller where you want extensions to be parsed correctly.
We then created a listing()
action in our controller, which serves a list of books from the database for our JSON format. Here you'll notice our use of a _serialize
key in our call to set()
. This is a special view variable that defines other view variables to be serialized for use in data views. The value of _serialize
can be a string, as the name of another view variable, or an array of various view variables to use. All variables defined for _serialize
must also be defined using the set()
method in the controller.
Internally, with the json
extension defined to be parsed with Router::parseExtensions()
, on every request with a .json
URL extension or with the Accept
header set to application/json
, the framework will switch the standard view class for JsonView
. This then handles the correct encoding and display of the JSON format.
See also
- Example usage of the Hash class is described at http://book.cakephp.org/2.0/en/core-utility-libraries/hash.html.
- More details on request handling can be found at http://book.cakephp.org/2.0/en/core-libraries/components/request-handl