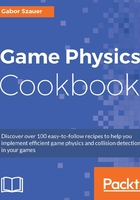
Cofactor
To get a cofactor of matrix, you first need to find the matrix of minor for that matrix. Given matrix M, find the cofactor of element and multiply the minor of that element by -1 raised to the
power:

Getting ready
We're going to create a generic function that will find the matrix of cofactors for any sized matrix, given the matrix of minors. We're going to call this generic Cofactor
function from more specific Cofactor
functions for 2 X 2 and 3 X 3 matrices.
How to do it…
Follow these steps to implement a generic cofactor function which will work on matrices of any size. We will use this generic function to implement the specific two and three dimensional square matrix cofactor functions:
- Declare all versions of the
Cofactor
function inmatrices.h
:void Cofactor(float* out, const float* minor, int rows, int cols); mat3 Cofactor(const mat3& mat); mat2 Cofactor(const mat2& mat);
- Implement the generic
Cofactor
function inmatrices.cpp
:void Cofactor(float* out, const float* minor, int rows, int cols) { for (int i = 0; i < rows; ++i) { for (int j = 0; j < cols; ++j) { int t = cols * j + i; // Target index int s = cols * j + i; // Source index float sign = powf(-1.0f, i + j); // + or – out[t] = minor[s] * sign; } } }
- Implement the 2 X 2 and 3 X 3
Cofactor
function inmatrices.cpp
. These functions just call the genericCofactor
function with the proper arguments:mat2 Cofactor(const mat2& mat) { mat2 result; Cofactor(result.asArray, Minor(mat).asArray, 2, 2); return result; } mat3 Cofactor(const mat3& mat) { mat3 result; Cofactor(result.asArray, Minor(mat).asArray, 3, 3); return result; }
How it works…
If we calculate the value of for every element of a matrix, you will notice it creates a checkered pattern. This is because a negative number to an even power results in a positive number, where a negative number to an odd power remains negative:

An easy way to remember how to calculate the cofactor matrix is to apply this checkered positive/negative pattern to the matrix of minors.