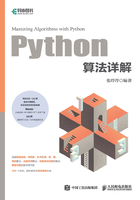
上QQ阅读APP看书,第一时间看更新
4.2.3 实践演练——在链表中增加比较功能
判断元素是否相等的操作符是“==”,我们可以使用此操作符定义单链表的一个相等比较函数。在下面的实例文件bijiao.py中,基于本章上一个范例增加比较操作函数,并且基于字典序的概念为链表定义大于、小于、大于或等于、小于或等于的判断功能。文件bijiao.py的主要实现代码如下所示。
源码路径:daima\第4章\bijiao.py
class LList: """ 省略已实现部分 """ #根据索引获得该位置的元素 def __getitem__(self, key): if not isinstance(key, int): raise TypeError if 0<=key<len(self): p = self._head num = -1 while p: num += 1 if key == num: return p.elem else: p = p.next else: raise IndexError #判断两个列表是否相等 def __eq__(self, other): #两个都为空列表,则相等 if len(self)==0 and len(other)==0: return True #两个列表中的元素个数相等,当每个元素都相等的情况下,两个列表相等 elif len(self) == len(other): for i in range(len(self)): if self[i] == other[i]: pass else: return False #全部遍历完后,两个列表相等 return True #两个列表中的元素个数不相等,返回Fasle else: return False #判断两个列表是否不相等 def __ne__(self, other): if self.__eq__(other): return False else: return True # 判断一个列表是否大于另一个列表 def __gt__(self, other): l1 = len(self) l2 = len(other) if not isinstance(other, LList): raise TypeError # 1.len(self) = len(other) if l1 == l2: for i in range(l1): if self[i] == other[i]: continue elif self[i] < other[i]: return False else: return True #遍历完都相等的话,说明两个列表相等,所以返回False return False # 2.len(self) > len(other) if l1 > l2: for i in range(l2): if self[i] == other[i]: continue elif self[i] < other[i]: return False else: return True #遍历完毕,前面的元素全部相等,则列表中元素个数多的一方大 #if self[l2-1] == other[l2-1]: return True # 3.len(self) < len(other) if l1 < l2: for i in range(l1): if self[i] == other[i]: continue elif self[i] < other[i]: return False else: return True #遍历完毕,前面的元素全部相等,则列表中元素个数多的一方大 #if self[l2-1] == other[l2-1]: return False # 判断一个列表是否小于另一个列表 def __lt__(self, other): #列表相等情况下,>会返回False,<在这里的判断会返回True,有错误。所以要考虑在相等的情况下也为False if self.__gt__(other) or self.__eq__(other): return False else: return True # 判断一个列表是否大于或等于另一个列表 def __ge__(self, other): """ if self.__eq__(other) or self.__gt__(other): return True else: return False """ #大于或等于和小于是完全相反的,所以可以依靠小于实现 if self.__lt__(other): return False else: return True # 判断一个列表是否小于或等于另一个列表 def __le__(self, other): """ if self.__eq__(other) or self.__lt__(other): return True else: return False """ ##小于或等于和大于是完全相反的,所以可以依靠大于实现 if self.__gt__(other): return False else: return True if __name__=="__main__": mlist1 = LList() mlist2 = LList() mlist1.append(1) mlist2.append(1) mlist1.append(2) mlist2.append(2) #mlist1.append(2) mlist2.append(6) mlist2.append(11) mlist2.append(12) mlist2.append(14) mlist1.printall() mlist2.printall() print(mlist1 == mlist2) print(mlist1 != mlist2) print(mlist1 <= mlist2) print(mlist2.__getitem__(1)) print(mlist1.__ne__(mlist2))
执行后会输出:
1, 2 1, 2, 6, 11, 12, 14 False True True 2 True