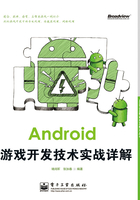
4.7 使用图形变换类Matrix
类Matrix的完整形式是Android.Graphics.Matrix,此类的功能是实现图形的变换操作,如常见的缩放和旋转处理。类Matrix有很多“成名绝技”,其中最著名的是如下几种常用的方法。
(1)void reset():重置一个Matrix对象;
(2)void set(Matrix src):复制一个源矩阵,和本类的构造方法Matrix(Matrix src)一样;
(3)boolean isIdentity():返回这个矩阵是否定义(已经有意义);
(4)void setRotate(float degrees):指定一个角度,以(0,0)为坐标进行旋转;
(5)void setRotate(float degrees, float px, float py):指定一个角度,以(px,py)为坐标进行旋转;
(6)void setScale(float sx, float sy):缩放处理;
(7)void setScale(float sx, float sy, float px, float py):以坐标(px, py)进行缩放;
(8)void setTranslate(float dx, float dy):平移;
(9)void setSkew (float kx, float ky, float px, float py:以坐标(px, py)进行倾斜;
(10)void setSkew (float kx, float ky):倾斜处理。
实例4-5 使用Matrix类实现图片缩放功能(daima\4\MatrixCH)。
本实例的实现流程如下所示。
step 1 编写布局文件main.xml,主要代码如下。
<?xml version="1.0" encoding="utf-8"?> <AbsoluteLayout android:id="@+id/layout1" android:layout_width="fill_parent" android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android" > <ImageView android:id="@+id/myImageView" android:layout_width="200px" android:layout_height="150px" android:src="@drawable/suofang" android:layout_x="0px" android:layout_y="0px" > </ImageView> <Button android:id="@+id/myButton1" android:layout_width="90px" android:layout_height="60px" android:text="@string/str_button1" android:textSize="18sp" android:layout_x="20px" android:layout_y="372px" > </Button> <Button android:id="@+id/myButton2" android:layout_width="90px" android:layout_height="60px" android:text="@string/str_button2" android:textSize="18sp" android:layout_x="210px" android:layout_y="372px" > </Button> </AbsoluteLayout>
step 2 编写主程序文件MatrixCH.java,实现图片缩放,缩小按钮响应mButton01.setOnClick-Listener,放大按钮响应mButton02.setOnClickListener。文件MatrixCH.java的主要实现代码如下。
public class MatrixCH extends Activity { /* 相关变量声明 */ private ImageView mImageView; private Button mButton01; private Button mButton02; private AbsoluteLayout layout1; private Bitmap bmp; private int id=0; private int displayWidth; private int displayHeight; private float scaleWidth=1; private float scaleHeight=1; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); /* 载入main.xml Layout */ setContentView(R.layout.main); /* 取得屏幕分辨率大小 */ DisplayMetrics dm=new DisplayMetrics(); getWindowManager().getDefaultDisplay().getMetrics(dm); displayWidth=dm.widthPixels; /* 屏幕高度需扣除下方Button高度 */ displayHeight=dm.heightPixels-80; /* 初始化相关变量 */ bmp=BitmapFactory.decodeResource(getResources(), R.drawable.suofang); mImageView = (ImageView)findViewById(R.id.myImageView); layout1 = (AbsoluteLayout)findViewById(R.id.layout1); mButton01 = (Button)findViewById(R.id.myButton1); mButton02 = (Button)findViewById(R.id.myButton2); /* 缩小按钮onClickListener */ mButton01.setOnClickListener(new Button.OnClickListener() { @Override public void onClick(View v) { small(); } }); /* 放大按钮onClickListener */ mButton02.setOnClickListener(new Button.OnClickListener() { @Override public void onClick(View v) { big(); } }); } /* 图片缩小的method */ private void small() { int bmpWidth=bmp.getWidth(); int bmpHeight=bmp.getHeight(); /* 设置图片缩小的比例 */ double scale=0.8; /* 计算出这次要缩小的比例 */ scaleWidth=(float) (scaleWidth*scale); scaleHeight=(float) (scaleHeight*scale); /* 产生reSize后的Bitmap对象 */ Matrix matrix = new Matrix(); matrix.postScale(scaleWidth, scaleHeight); Bitmap resizeBmp = Bitmap.createBitmap(bmp,0,0,bmpWidth, bmpHeight,matrix,true); if(id==0) { /* 如果是第一次按,就删除原来默认的ImageView */ layout1.removeView(mImageView); } else { /* 如果不是第一次按,就删除上次放大缩小所产生的ImageView */ layout1.removeView((ImageView)findViewById(id)); } /* 产生新的ImageView,放入reSize的Bitmap对象,再放入Layout中 */ id++; ImageView imageView = new ImageView(suofang.this); imageView.setId(id); imageView.setImageBitmap(resizeBmp); layout1.addView(imageView); setContentView(layout1); /* 因为图片放到最大时放大按钮会消失,所以在缩小时把它重设为enable */ mButton02.setEnabled(true); } /* 图片放大的method */ private void big() { int bmpWidth=bmp.getWidth(); int bmpHeight=bmp.getHeight(); /* 设置图片放大的比例 */ double scale=1.25; /* 计算这次要放大的比例 */ scaleWidth=(float)(scaleWidth*scale); scaleHeight=(float)(scaleHeight*scale); /* 产生reSize后的Bitmap对象 */ Matrix matrix = new Matrix(); matrix.postScale(scaleWidth, scaleHeight); Bitmap resizeBmp = Bitmap.createBitmap(bmp,0,0,bmpWidth, bmpHeight,matrix,true); if(id==0) { /* 如果是第一次按,就删除原来设置的ImageView */ layout1.removeView(mImageView); } else { /* 如果不是第一次按,就删除上次放大缩小所产生的ImageView */ layout1.removeView((ImageView)findViewById(id)); } /* 产生新的ImageView,放入reSize的Bitmap对象,再放入Layout中 */ id++; ImageView imageView = new ImageView(suofang.this); imageView.setId(id); imageView.setImageBitmap(resizeBmp); layout1.addView(imageView); setContentView(layout1); /* 如果再放大会超过屏幕大小,就让Button消失*/ if(scaleWidth*scale*bmpWidth>displayWidth|| scaleHeight*scale*bmpHeight>displayHeight) { mButton02.setEnabled(false); } }
执行后将显示一幅图片和两个按钮,如图4-5所示,分别单击“缩小”和“放大”按钮后会实现对图片的缩小、放大处理。

图4-5 执行效果